Debugging Network Requests Using Flutter HTTP Interceptor
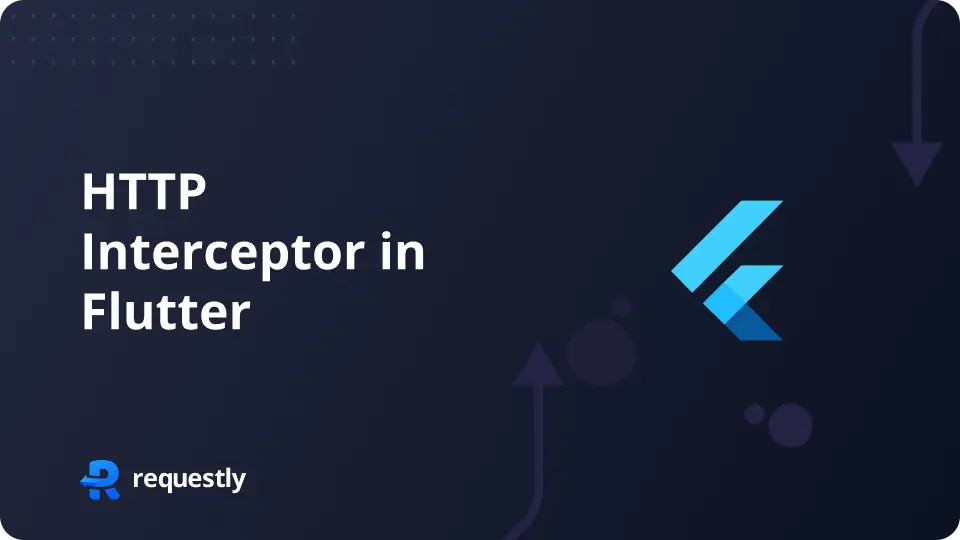
Introduction
When developing a Flutter application that interacts with a backend server, it’s essential to have a way to monitor and debug network requests. Flutter HTTP interceptor allow you to intercept and modify requests and responses, providing a powerful tool for debugging and logging.
Prerequisites
Before you start, make sure you have the following:
- Flutter SDK installed
- Basic knowledge of Dart and Flutter
- A Flutter project set up
Steps
Step 1: Add Dependencies
First, you need to add the necessary dependencies to your pubspec.yaml
file. You’ll need the http
package for making network requests and the http_interceptor
package for intercepting requests and responses.
dependencies:
flutter:
sdk: flutter
http: ^0.13.3
http_interceptor: ^1.0.2
Run the following command to install the dependencies:
flutter pub get
Step 2: Create an Interceptor
Create a custom interceptor to log the request and response details. This interceptor will print the request method, URL, headers, and body, as well as the response status, headers, and body.
Create a new file lib/network/logging_interceptor.dart
:
import 'package:http_interceptor/http_interceptor.dart';
class LoggingInterceptor implements InterceptorContract {
@override
Future interceptRequest({required RequestData data}) async {
print('Request:');
print('Method: ${data.method}');
print('URL: ${data.url}');
print('Headers: ${data.headers}');
print('Body: ${data.body}');
return data;
}
@override
Future interceptResponse({required ResponseData data}) async {
print('Response:');
print('Status: ${data.statusCode}');
print('Headers: ${data.headers}');
print('Body: ${data.body}');
return data;
}
}
Step 3: Set Up the HTTP Client with Interceptors
Create an HTTP client that uses the interceptor. This client will be used to make network requests in your application.
Create a new file lib/network/http_client.dart
:
import 'package:http/http.dart' as http;
import 'package:http_interceptor/http/intercepted_http.dart';
import 'package:projectName/network/logging_interceptor.dart';
final client = InterceptedHttp.build(
interceptors: [LoggingInterceptor()],
requestTimeout: Duration(seconds: 10),
);
Step 4: Use the Intercepted HTTP Client
Use the intercepted HTTP client to make network requests. This example fetches data from a sample API.
Create a new file lib/network/api_service.dart
:
import 'dart:convert';
import 'package:projectName/network/http_client.dart';
Future fetchData() async {
final response = await client.get(Uri.parse('https://jsonplaceholder.typicode.com/todos/1'));
if (response.statusCode == 200) {
final data = jsonDecode(response.body);
print('Data: $data');
} else {
print('Failed to load data');
}
}
Step 5: Integrate with Your Flutter App
Integrate the fetchData
function into your Flutter app, for example, in a button’s onPressed
callback.
Update your lib/main.dart
file:
import 'package:flutter/material.dart';
import 'package:projectName/network/api_service.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('HTTP Interceptor Example'),
),
body: Center(
child: ElevatedButton(
onPressed: fetchData,
child: Text('Fetch Data'),
),
),
),
);
}
}
Step 6: Run the app
Connect your device or start an emulator, then run the following command to start the application:
flutter run
LoggingInterceptor
.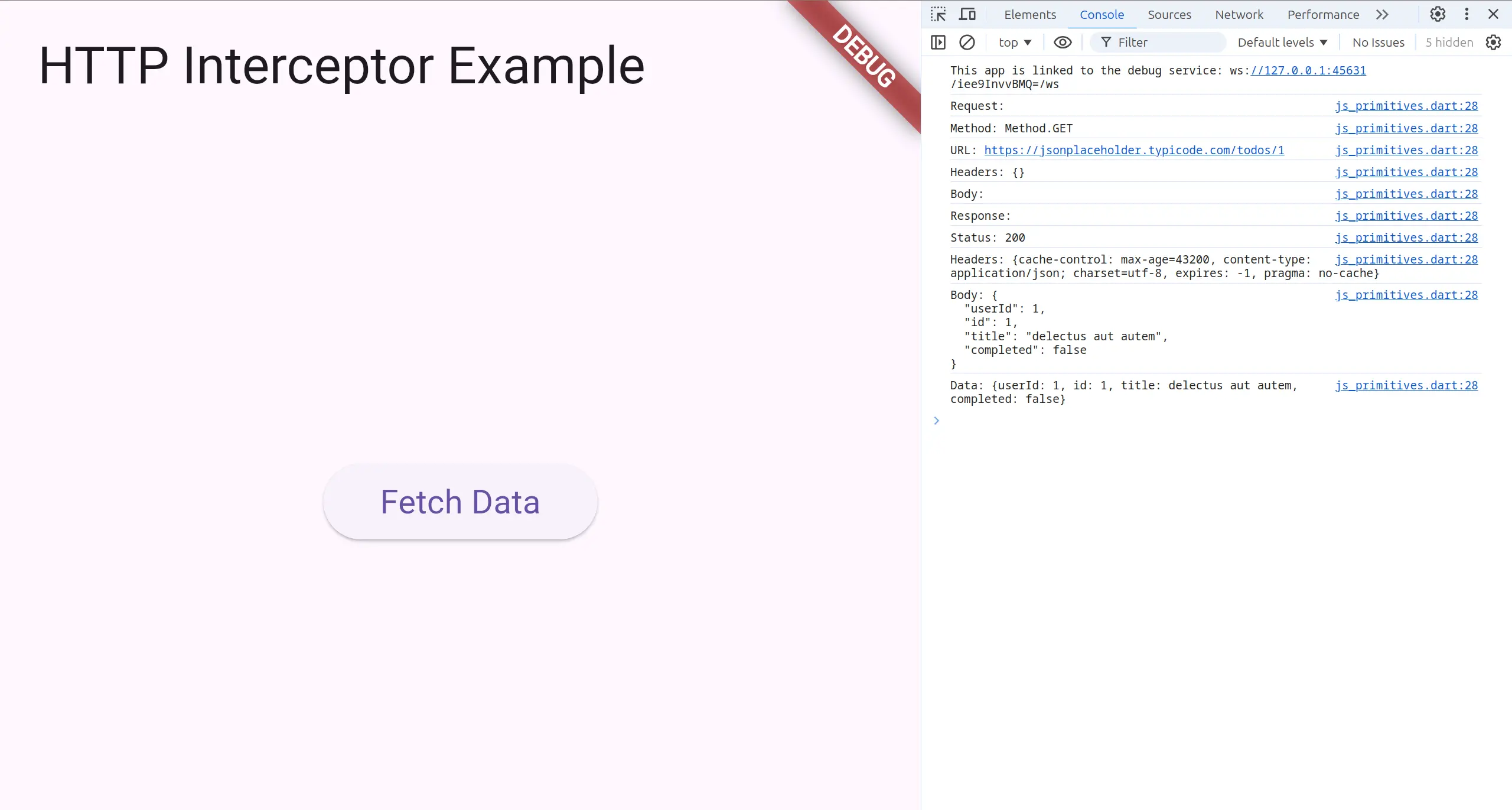
A Better HTTP Interceptor for Flutter
Requestly is a powerful HTTP Interceptor built for teams.
Speed up local development by Intercepting & Modifying HTTP Requests
Emulate those tough edge cases by simply modifying headers and body of responses.
Switch environments by redirecting your API calls using Replace rule.
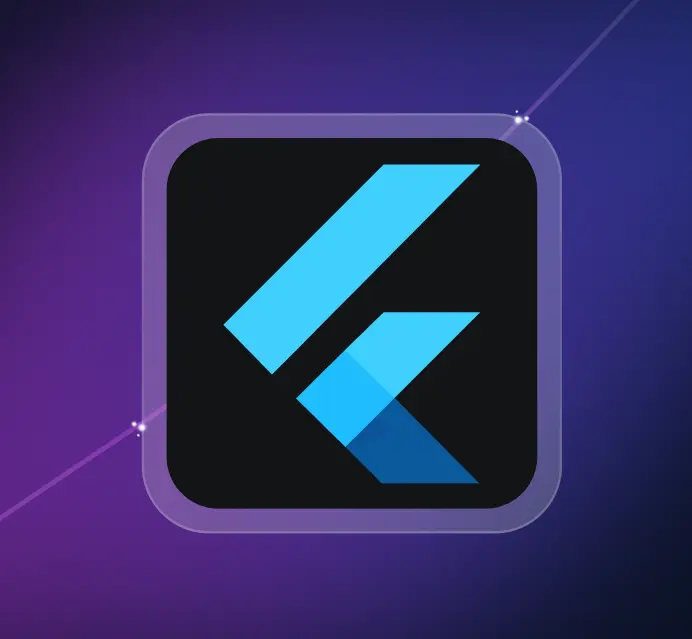
Conclusion
Using Flutter HTTP interceptors is a powerful way to debug network requests and responses. By setting up a custom interceptor, you can log detailed information about each request and response, making it easier to identify and fix issues in your application. This approach can significantly improve the reliability and maintainability of your Flutter projects.
Contents
Subscribe for latest updates
Share this article
Related posts
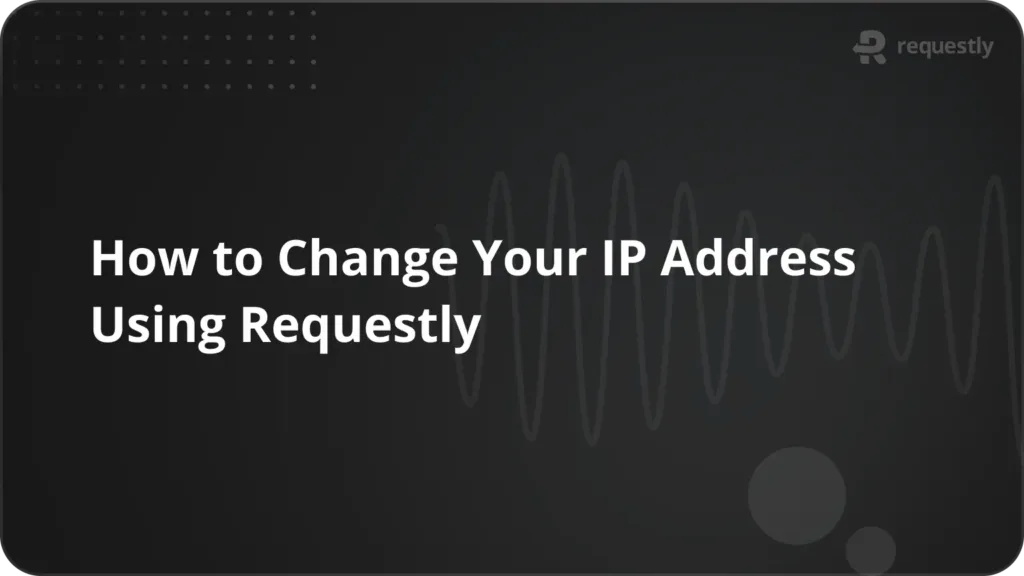
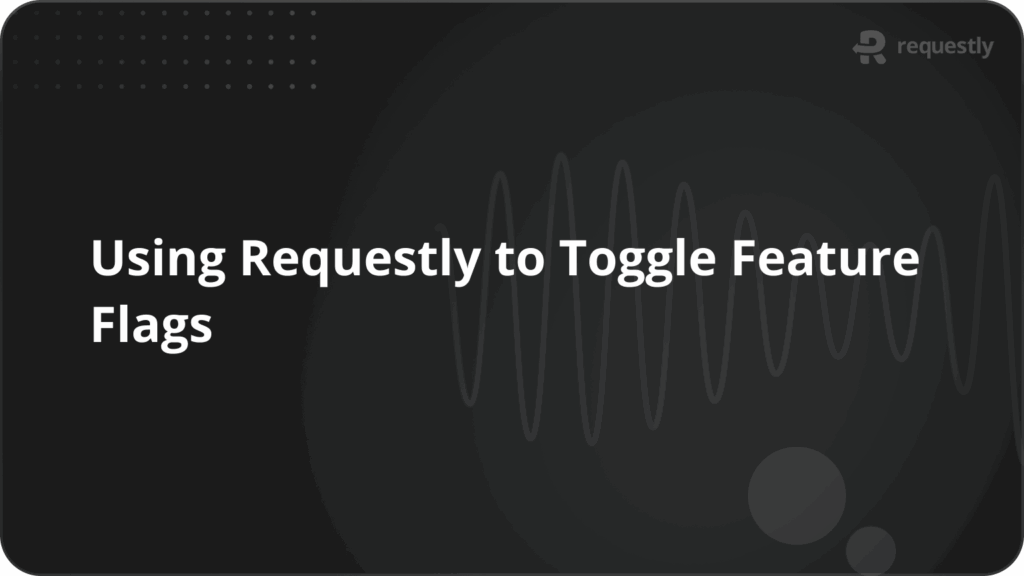
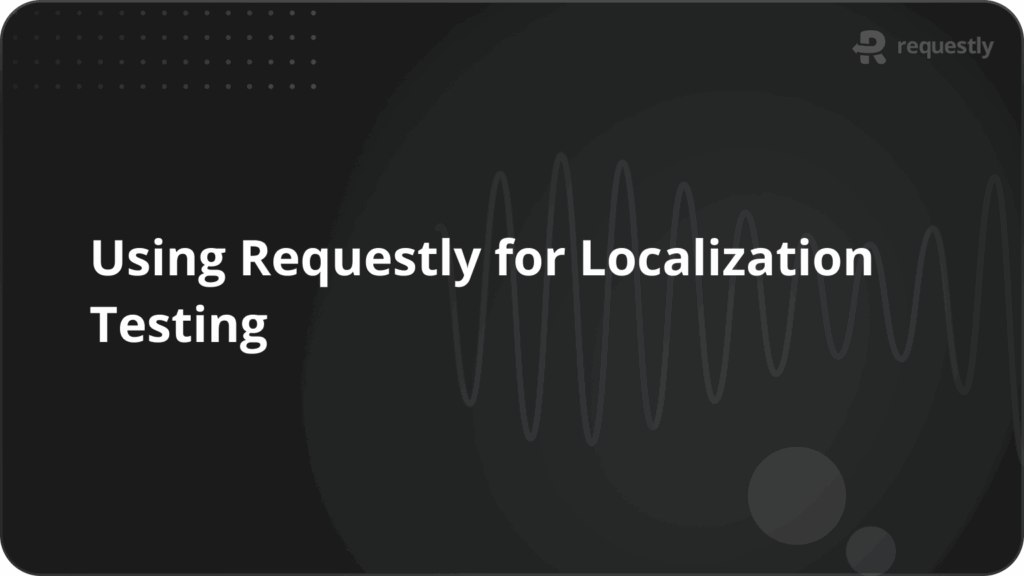