How to use access token from another API response
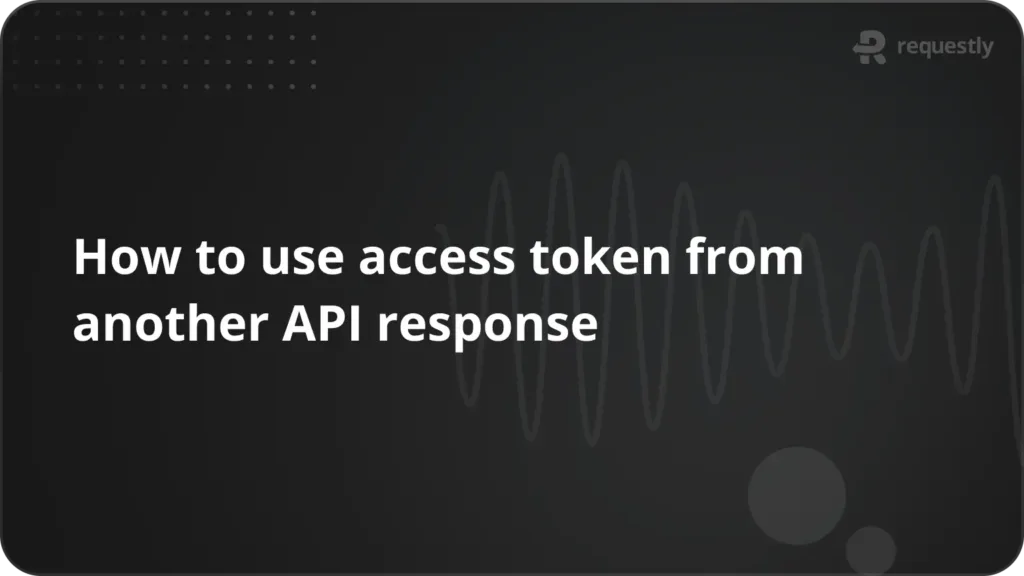
Introduction
When working with APIs in API clients, authentication tokens are often used for testing multiple endpoints. Typically, an auth token is requested from the server in one request and then used to perform various operations across different endpoints.
A common challenge is handling token expiration and reusability without manually copying and pasting the token each time it refreshes. A Stack Overflow question highlights this issue.
Instead of manually copying and pasting the token every time it refreshes, you can use variables and authorization in Requestly to apply the same authentication across all requests in a collection.
Using Authentication in Requestly
Let’s say we’re working with a mock API that provides an authentication endpoint:
Requesting an Auth Token
We first send a POST request to the authentication endpoint to obtain an access token.
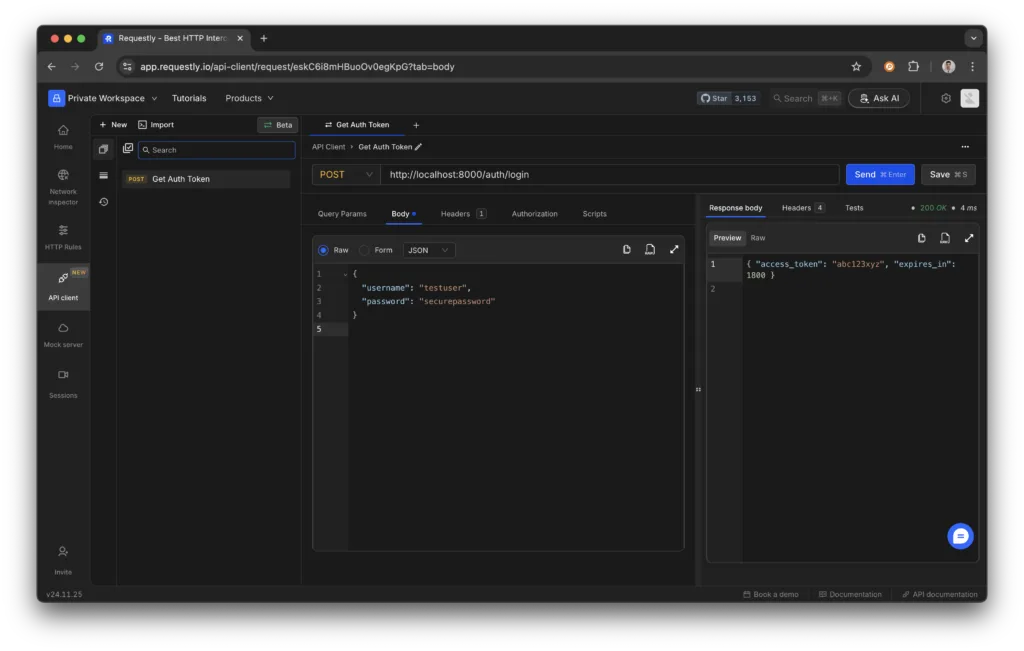
{
"access_token": "",
"expires_in": 1800
}
Extracting and Storing the Token
We use a post-response script to extract the access_token from the response and save it in an environment variable:
const authToken = JSON.parse(rq.response.body)['access_token'];
rq.environment.set("authToken", authToken);
Using the Stored Token for API Requests
Now that we’ve stored the token, we can use it in subsequent API requests. Instead of manually copying the token, we reference it dynamically in our requests.
Case 1: Setting a Collection Authorization
If you’re working with multiple requests in a collection, you can set an Authorization at the collection level. This ensures that all requests in the collection automatically include authentication without needing to manually add it to each request.
📖 To learn more about authorization in Requestly, refer to the official documentation.
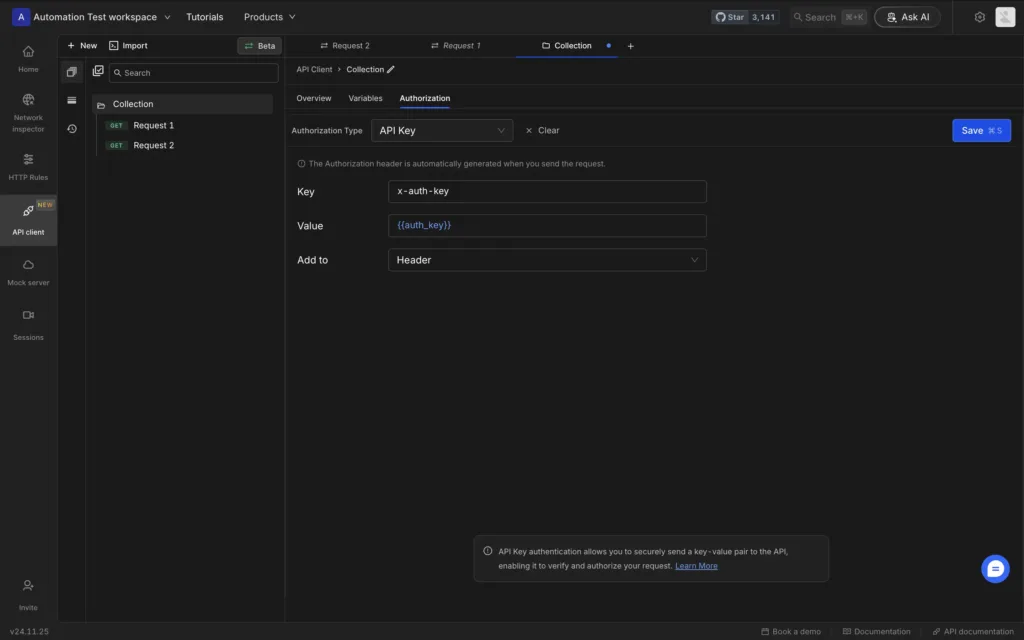
Case 2: Using variables in Request
You can reference any stored environment variable using the {{variable_name}} syntax in:
- Request Headers (e.g.,
Authorization: Bearer {{authToken}}
) - Query Parameters (e.g.,
?token={{authToken}}
) - Request Body (for JSON payloads that require an access token)
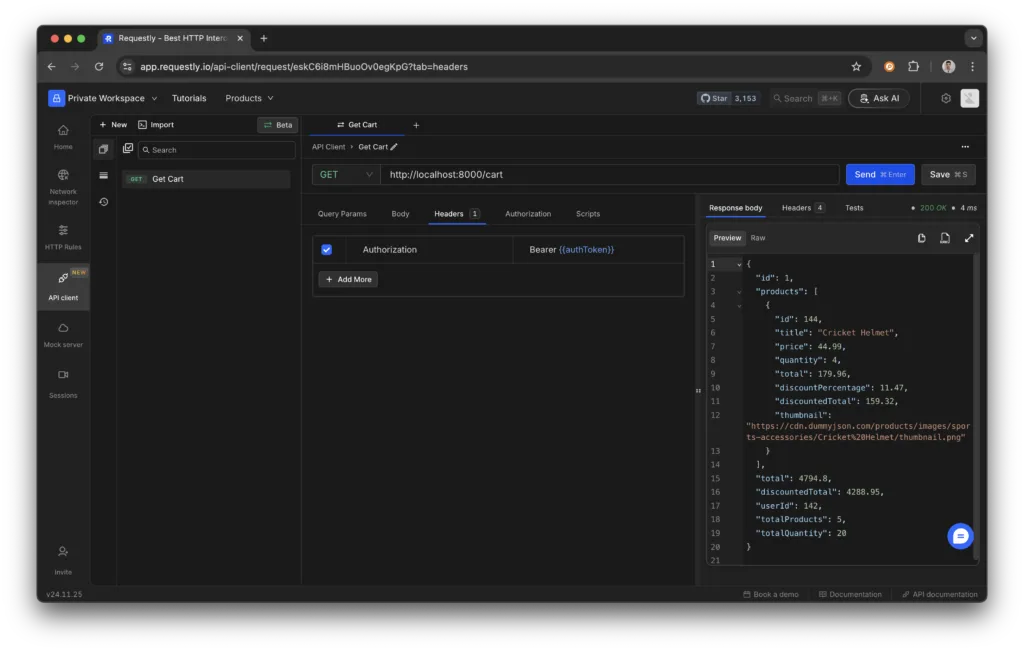
Case 3: Using Variables in Pre/Post Scripts
For more advanced use cases, you can retrieve an environment variable in pre-request or post-response scripts using:
const token = rq.environment.get("authToken");
This is useful when you need to modify or validate the token before sending the request.
Conclusion
Automating authentication in Requestly’s API Client saves time and ensures seamless API testing. By storing tokens as environment variables and using collection-level authorization, you can avoid manual updates and streamline workflows.
Contents
Subscribe for latest updates
Share this article
Related posts
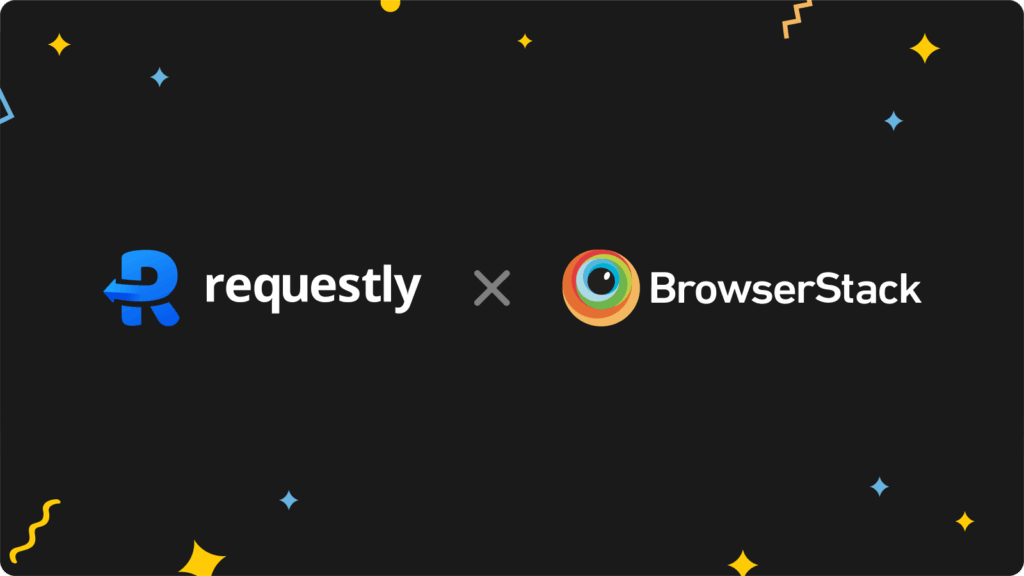
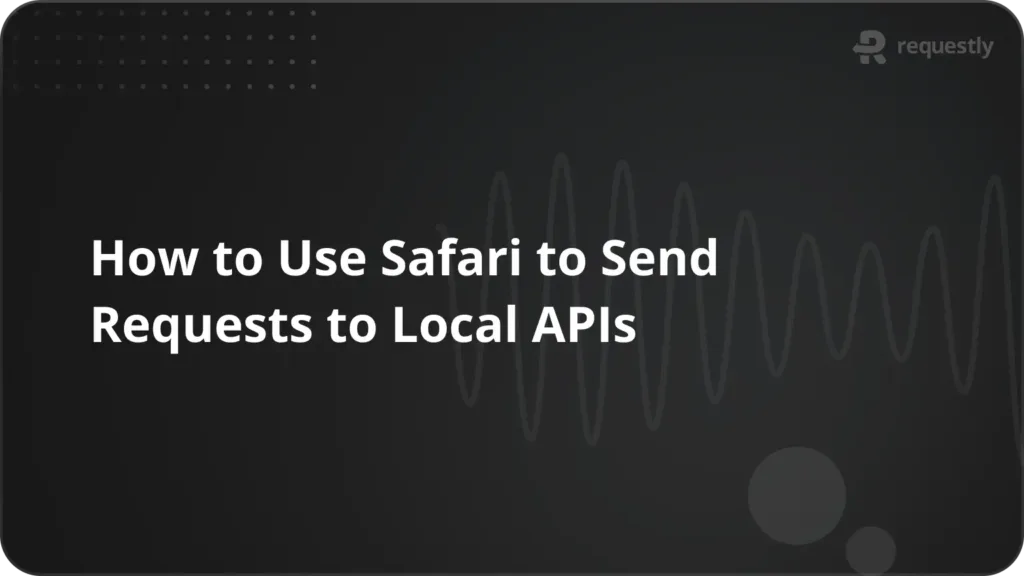
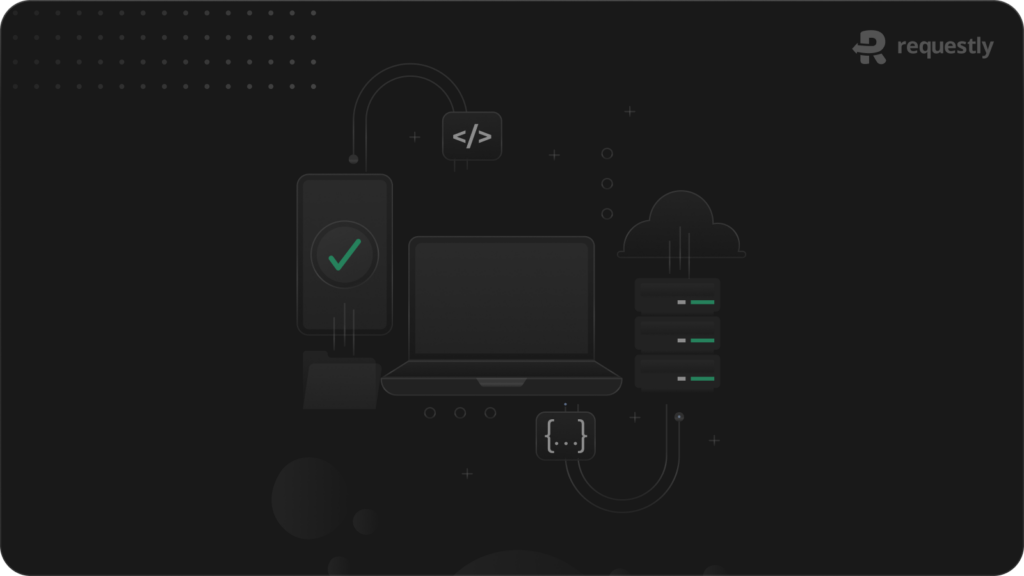