What are API Headers and Body ?
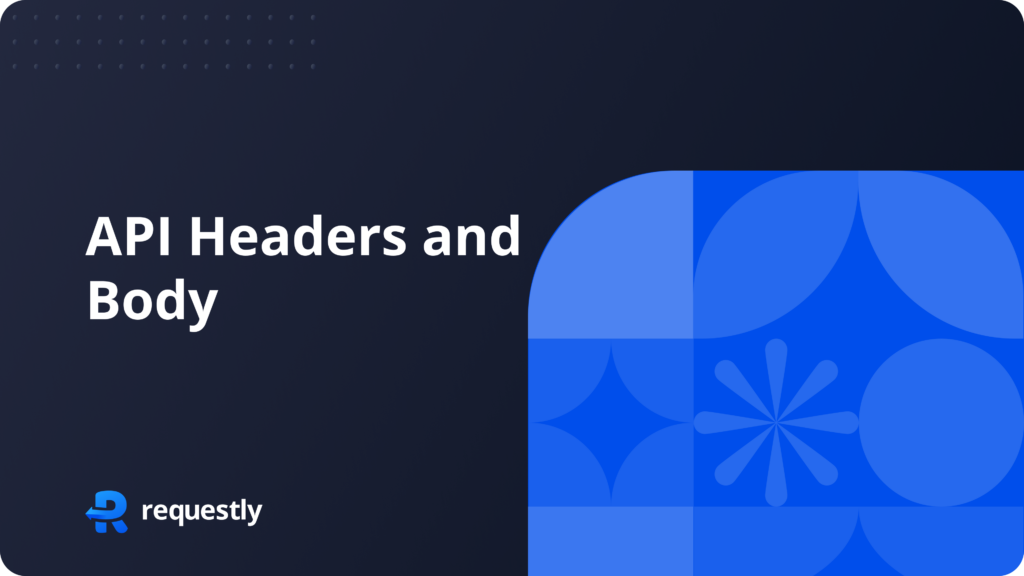
Introduction:
API stands for Application Programming Interface. It is a set of rules and protocols that allows different software applications to communicate and interact with each other. APIs act as intermediaries, enabling different systems to request and exchange data, services, or functionalities seamlessly. Key elements include API headers and body, which structure and manage these interactions efficiently.
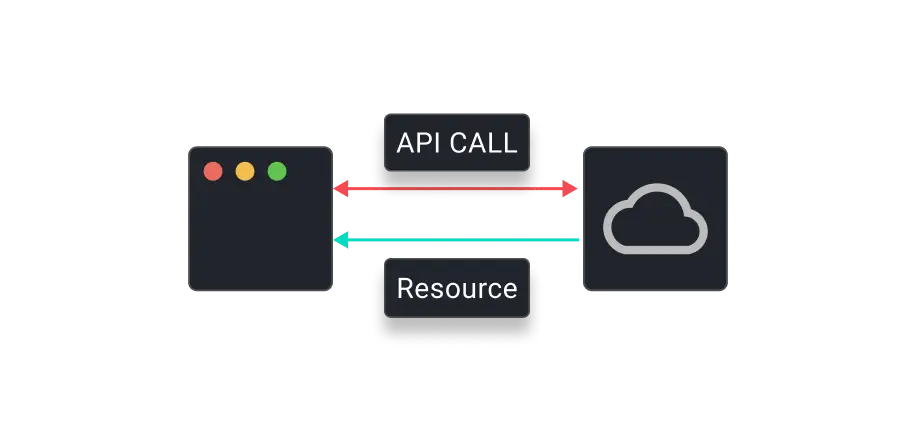
They play a crucial role in modern software development, facilitating integration, scalability, and interoperability across various applications and platforms.
The role of APIs in modern software development can be summarized as follows:
- Data Access and Integration: APIs enable applications to access and retrieve data from external sources, databases, or other applications. They facilitate data integration and synchronization, allowing applications to work with data from multiple sources efficiently.
- Cross-Platform Compatibility: APIs promote cross-platform compatibility, allowing applications to run on different operating systems, devices, or browsers. Developers can build applications that work on various platforms by leveraging APIs with consistent interfaces.
- Scalability and Microservices: APIs are a fundamental component of microservices architecture. By breaking down monolithic applications into smaller, independent services, APIs allow developers to scale and deploy specific services independently.
- Third-Party Integrations: APIs enable seamless integration with third-party services and platforms. This empowers developers to leverage specialized functionalities and services without building them from scratch, thus accelerating the development process.
- Easier Software Maintenance: With APIs, developers can update or replace underlying components or services without affecting the applications that use them, simplifying software maintenance and upgrades.
Understanding the Key Components of an API Request
An API request typically consists of several components that convey specific information to the server. These components help the client and the server understand and fulfill each other’s requirements.
We will use popular Github API to explain the API components.
API Endpoint
The API endpoint is the URL that identifies the resource or action you want to access. In this case, we want to retrieve information about a user, so the endpoint is the GitHub API’s user API endpoint. API Endpoint: https://api.github.com/users/{username}/events?type=public
Replace {username}
with the GitHub username of the user you want to fetch information for.
HTTP Method
Since we want to retrieve data, we’ll use the HTTP GET method. Common HTTP methods include:
- GET: Requests data from the server (read-only operation).
- POST: Sends data to the server to create a new resource.
- PUT: Updates an existing resource with new data.
- DELETE: Deletes a resource from the server.
Request Headers
API headers are key-value pairs sent between the client and server in an HTTP request or response. They provide important metadata about the request or response, such as the content type, authorization credentials, and more.
An API request typically consists of several components that convey specific information to the server. These components help the client and the server understand and fulfill each other’s requirements.
Purpose and Significance
Headers play a critical role in the functioning of APIs by:
- Providing information about the request or response
- Controlling caching and other behaviors
- Enhancing security through authentication mechanisms
Common Types of API Headers
Authorization: Used to pass authentication information. Examples include Bearer tokens and Basic auth.
Authorization: Bearer
Content-Type: Specifies the media type of the resource being sent
Content-Type: application/json
Cache-Control: Controls how and for how long the response is cached
Cache-Control: no-cache
We can use number of directives for cache control, such as:
- public – A cache can store the response.
- private – The response can be stored, but not in a shared cache.
- max-age – The time in seconds for which a cached response is acceptable.
- no-cache – Used by clients to specify that a stored response shouldn’t be used, or by the server shouldn’t use the response for subsequent requests without validation on the origin server.
- no-store – A cache must not store the request or response – if specified by the client or server respectively.
User-Agent: Identifies the client software making the request.
User-Agent: Mozilla/5.0
Custom Headers: Developers can create custom headers to pass specific information.
X-Custom-Header: customValue
API Body
The API body is the part of the request or response that contains the actual data being transferred. It is particularly important in methods such as POST, PUT, PATCH, and DELETE, where the client needs to send data to the server.
Types of Data in API Bodies
JSON: The most common format used in APIs today. It is lightweight and easy to parse.
{
"data": {
"user": {
"name": "John",
"age":30
}
}
}
- XML: Another format used, especially in older APIs or those requiring more structured data.
John
30
Conclusion
Understanding API headers and body is essential for any developer working with APIs. Headers provide vital information and control various aspects of the communication, while the body contains the actual data being exchanged. By following best practices, you can ensure efficient and secure API interactions.
Contents
Subscribe for latest updates
Share this article
Related posts
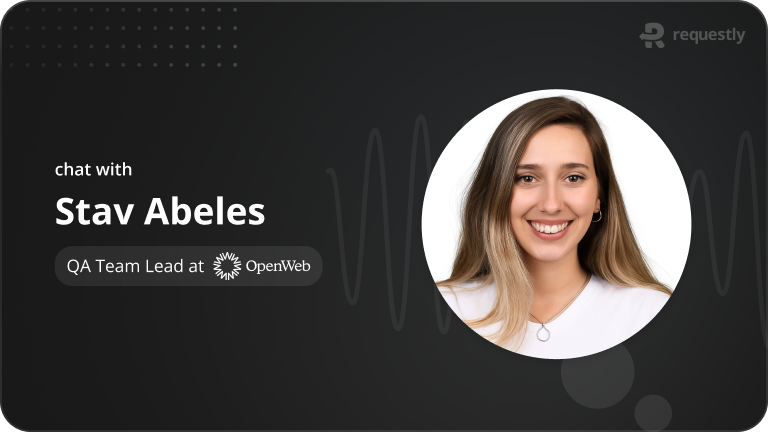
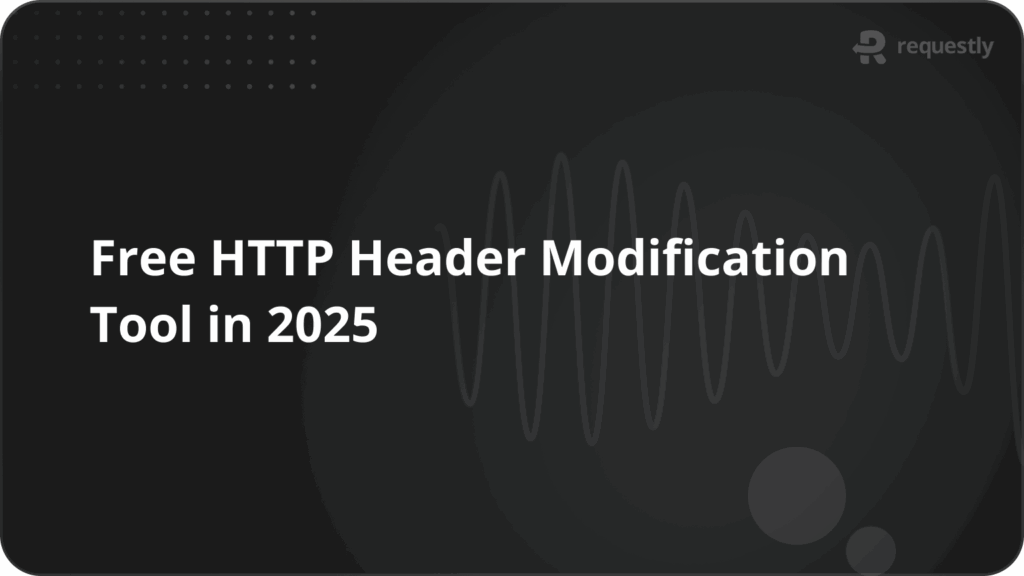
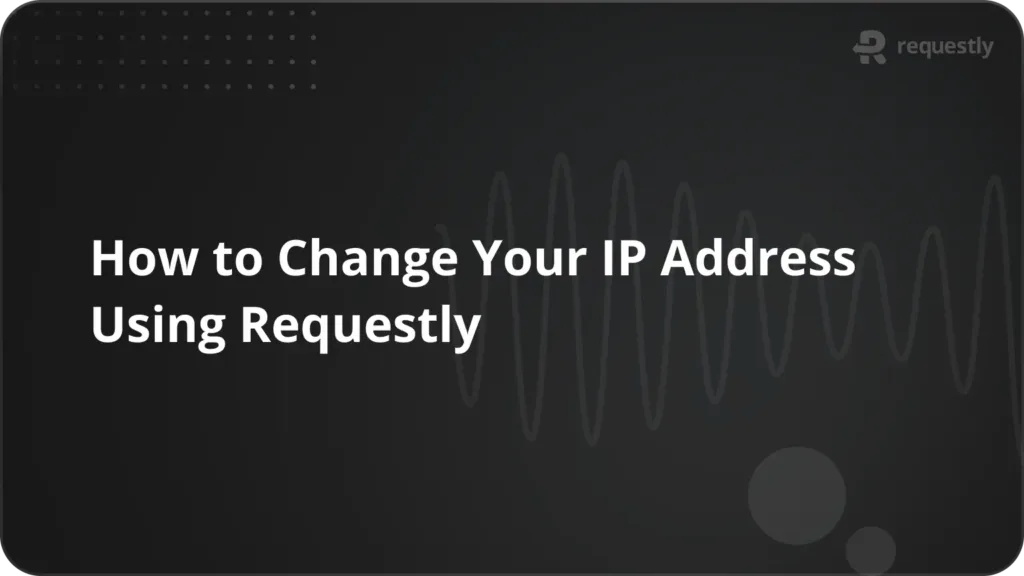