How to implement Axios Request Interceptors in Next.js
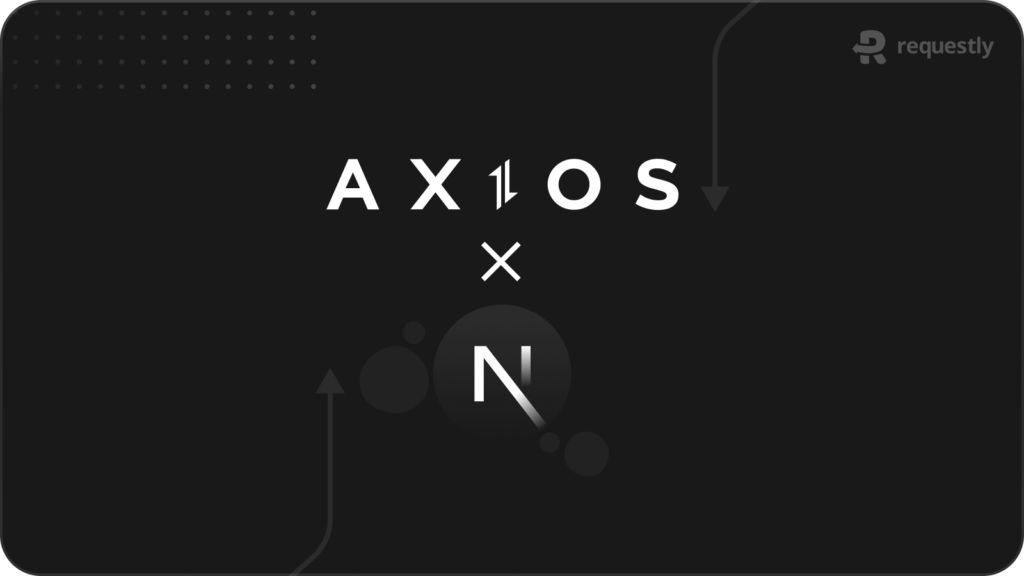
Introduction
Axios is a widely used JavaScript library that makes it easier to send HTTP requests to servers. One of its standout features is the interceptor, which allows our app to catch requests and responses. Axios interceptors let us set up functions that run for each request or response before they reach the application. This is helpful for tasks like adding authentication tokens, logging, and handling errors globally, making our code cleaner and easier to manage.
In this blog post, we’ll learn how to implement Axios request interceptors in a Next.js application. We’ll start by setting up Axios, and then we’ll see how to create and use request and response interceptors. By the end, you’ll know how to use interceptors to improve your application and keep your code organized.
Setup the Project
Before diving into how to implement Axios request interceptors in a Next.js application, make sure you have the following:
Node.js and npm/yarn Installed: Ensure you have Node.js and npm (or yarn) installed on your machine. You can download Node.js from here.
A Next.js Project Setup: You should have a Next.js project setup. If you don’t have one, you can create a new Next.js project using Create Next App:
npx create-next-app my-axios-app
cd my-axios-app
npm install axios
or
yarn add axios
Implementing Request Interceptors
Request interceptors in Axios let you modify requests before they reach the server. They’re useful for adding authentication tokens, setting custom headers, or logging requests. Here’s how to implement Axios request interceptors in a Next.js application.
Step 1: Create an Axios Instance
Create a new file axiosInstance.js
in the lib
folder (or any preferred location in your project). You can add a request interceptor to the Axios instance you created earlier. This interceptor will be executed before every request is sent out.
Creating an Axios instance allows you to set default configurations, such as the base URL and headers, that will be applied to all requests made with that instance. This helps in keeping your code DRY (Don’t Repeat Yourself).
Create a new file named axiosInstance.js
in your lib
folder and set up your Axios instance:
// lib/axiosInstance.js
import axios from 'axios';
const axiosInstance = axios.create({
baseURL: 'https://dummyjson.com', // Replace with your API base URL
timeout: 1000,
headers: { 'Content-Type': 'application/json' }
});
// Add a request interceptor
axiosInstance.interceptors.request.use(
function (config) {
// Do something before the request is sent
// For example, add an authentication token to the headers
const token = localStorage.getItem('authToken'); // Retrieve auth token from localStorage
if (token) {
config.headers.Authorization = `Bearer ${token}`;
}
return config;
},
function (error) {
// Handle the error
return Promise.reject(error);
}
);
export default axiosInstance;
Here’s a summary of what we’ve done:
- Created an Axios instance using axios.create().
- Set the
baseURL
to the base URL of your API. You can adjust this to match your API’s configuration. - Used interceptors.request.use() to intercept and modify outgoing requests. This allows us to add headers, authentication tokens, or make other changes to the request configuration.
Step 2: Use the Axios Instance in Next.js Pages or Components
With the request interceptor set up, you can use the Axios instance in your Next.js pages or components as usual. The interceptor will automatically add the token (or perform any other configured actions) before each request is sent.
// pages/index.js
import React, { useEffect, useState } from 'react';
import axiosInstance from '../lib/axiosInstance';
export default function Home() {
const [data, setData] = useState(null);
useEffect(() => {
axiosInstance.get('/products/1') // Replace with your API endpoint
.then(response => {
setData(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
}, []);
return (
Data from API
{data ? (
{JSON.stringify(data, null, 2)}
) : (Loading...
)} );
}
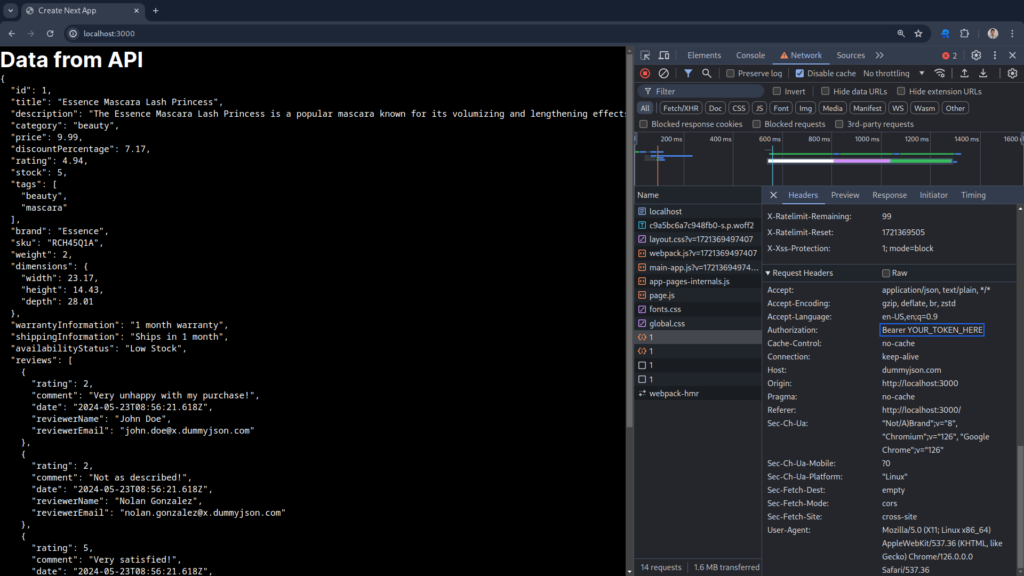
Step 3: Customizing the Interceptor
You can customize the request interceptor to perform other actions as needed. For example, you might want to log the details of each request:
axiosInstance.interceptors.request.use(
function (config) {
// Log the request details
console.log('Request:', config);
return config;
},
function (error) {
// Handle the error
return Promise.reject(error);
}
);
This setup will log the details of each request to the console, which can be helpful for debugging purposes.
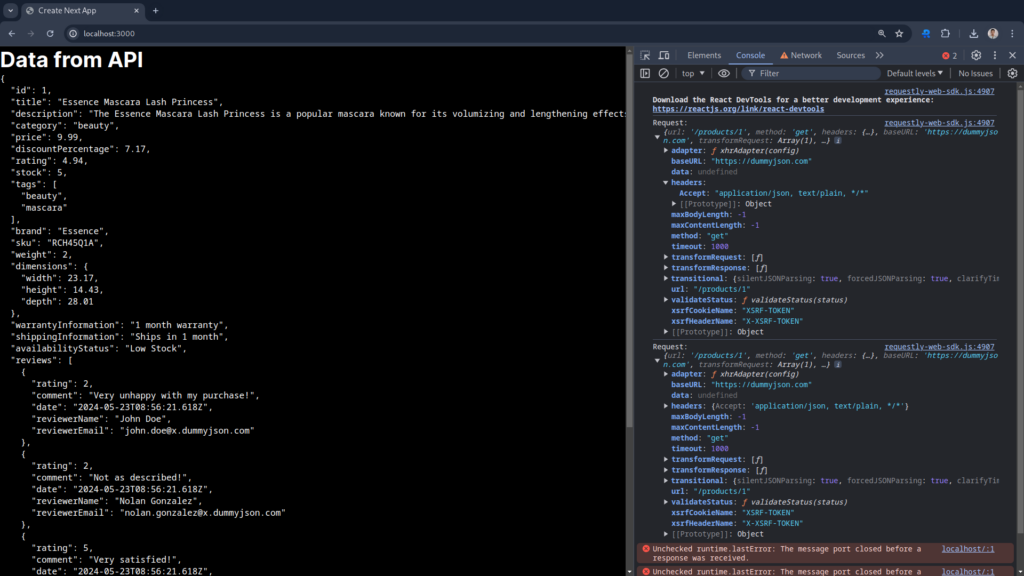
By implementing request interceptors in your Next.js application, you can ensure that all requests are consistently modified or enhanced before they are sent, improving the maintainability and functionality of your code.
Implementing Response Interceptors
Similar to how request interceptors allow you to modify outgoing requests, response interceptors in Axios enable you to manage responses globally before they reach your application code. This is especially helpful for tasks such as error handling, response transformation, and logging. Let’s explore how to implement response interceptors in a Next.js application using Axios.
Step 1: Create the Response Interceptor
In your axiosInstance.js
file, you can add a response interceptor to the Axios instance you created. This interceptor will be executed after every response is received.
// lib/axiosInstance.js
import axios from 'axios';
const axiosInstance = axios.create({
baseURL: 'https://dummyjson.com', // Replace with your API base URL
timeout: 1000,
headers: { 'Content-Type': 'application/json' }
});
// Add a request interceptor
axiosInstance.interceptors.request.use(
function (config) {
// Do something before the request is sent
const token = localStorage.getItem('authToken'); // Retrieve auth token from localStorage
if (token) {
config.headers.Authorization = `Bearer ${token}`;
}
return config;
},
function (error) {
// Handle the error
return Promise.reject(error);
}
);
// Add a response interceptor
axiosInstance.interceptors.response.use(
function (response) {
// Do something with the response data
console.log('Response:', response);
return response;
},
function (error) {
// Handle the response error
if (error.response && error.response.status === 401) {
// Handle unauthorized error
console.error('Unauthorized, logging out...');
// Perform any logout actions or redirect to login page
}
return Promise.reject(error);
}
);
export default axiosInstance;
Step 2: Use the Axios Instance in Next.js Pages or Components
With the response interceptor set up, you can use the Axios instance in your Next.js pages or components as usual. The interceptor will automatically handle responses and errors based on your configuration.
// pages/index.js
import { useEffect, useState } from 'react';
import axiosInstance from '../lib/axiosInstance';
export default function Home() {
const [data, setData] = useState(null);
useEffect(() => {
axiosInstance.get('/products/1') // Replace with your API endpoint
.then(response => {
setData(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
}, []);
return (
Data from API
{data ? (
{JSON.stringify(data, null, 2)}
) : (Loading...
)} );
}
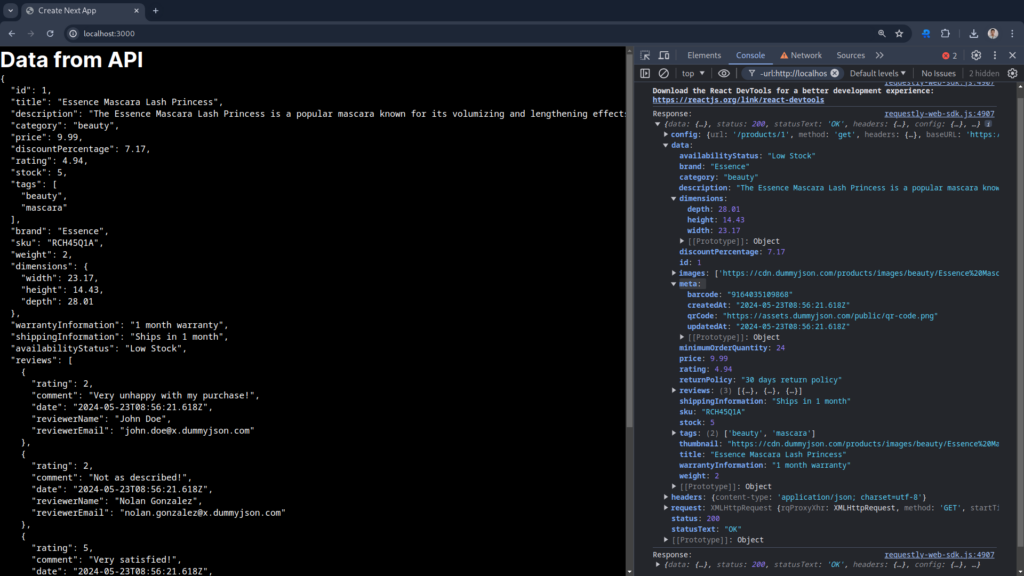
By implementing response interceptors in your Next.js application, you can centralize response handling, improving code maintainability and application robustness. Whether it’s logging, transforming data, or managing errors, response interceptors provide a powerful way to manage HTTP responses efficiently.
Framework-Independent Alternative: Using Requestly
While Axios has powerful tools for processing HTTP requests within applications, integrating and managing interceptors directly within your codebase can be difficult and demand changes to your application’s architecture. Instead of depending on framework-specific solutions such as Axios interceptors, developers can use Requestly, a browser extension that modifies network requests and responses without requiring any changes to the application’s code. This method has various advantages over standard interceptors:
Simplifying Modifications with Requestly
- No Code Changes Required: Unlike implementing interceptors in your application code, which requires understanding and modifying the codebase, Requestly operates entirely from the browser. This means developers can modify requests and responses dynamically without touching the application’s source code.
- Flexibility Across Technologies: Requestly’s framework-independent nature allows it to work seamlessly across different projects and technologies. Whether you’re working with React, Angular, Vue.js, or any other framework, Requestly provides a consistent interface for managing network traffic.
Advantages of Using Requestly
- Ease of Use: Requestly simplifies the process of modifying network requests and responses through an intuitive browser extension interface. This accessibility makes it ideal for developers of all skill levels, from beginners to advanced users.
- Immediate Testing and Debugging: With Requestly, developers can instantly test and debug different scenarios by altering headers, URLs, or response content. This capability speeds up development cycles and enhances troubleshooting efficiency.
- Enhanced Privacy and Security: Requestly empowers developers to block or modify requests to enhance privacy, security, and compliance with data protection regulations. For instance, blocking tracking scripts or adding secure headers can be done effortlessly.
Example Use Cases
- Modify Server Response: Modify response content to simulate various server behaviors without backend changes.
- Testing Different API Requests: Dynamically alter request to test different API endpoints or data payloads.
- Blocking Network Request : Test your website under scenarios where certain external resources are unavailable
- Adding Custom Headers : Add authentication tokens or custom CORS headers for testing APIs that require specific headers.
How to use Requestly Interceptor
Modify API Response
Requestly allows you to modify API responses. It provides a user-friendly interface for overriding the response body of API requests, allowing you to mimic different data scenarios that your frontend might encounter.
Insert/Inject Script
Insert/Inject Script Rule allows you to inject JavaScript and CSS into web pages as they load. This means you can modify the DOM, change styles, or even add new functionality without altering the source code directly. It’s important for testing hypotheses or debugging during the development and quality assurance process. Learn more about it here.
Replace Rule
Replace Rule enables you to replace a String in URL with another String. This feature is particularly useful for developers to swap the API endpoints from one environment to another or change something specific in the URL. Requests are matched with source condition, and find and replace
are performed on those requests by redirecting to the resulting URL. Learn more about this rule here.
Conclusion
In this blog post, we’ve explored the powerful concept of intercepting requests with Axios in a Next.js application. This allows developers to have more control over HTTP requests and responses within their applications. Whether it’s adding authentication tokens, logging requests for debugging purposes, or handling errors globally, Axios interceptors provide a flexible solution to meet diverse development needs.
If you like this blog check out our other blog on How to implement Axios interceptor in React
Contents
- Introduction
- Setup the Project
- Implementing Request Interceptors
- Step 1: Create an Axios Instance
- Step 2: Use the Axios Instance in Next.js Pages or Components
- Data from API
- Step 3: Customizing the Interceptor
- Implementing Response Interceptors
- Step 1: Create the Response Interceptor
- Step 2: Use the Axios Instance in Next.js Pages or Components
- Data from API
- Framework-Independent Alternative: Using Requestly
- Simplifying Modifications with Requestly
- Advantages of Using Requestly
- Example Use Cases
- How to use Requestly Interceptor
- Modify API Response
- Insert/Inject Script
- Replace Rule
- Conclusion
Subscribe for latest updates
Share this article
Related posts
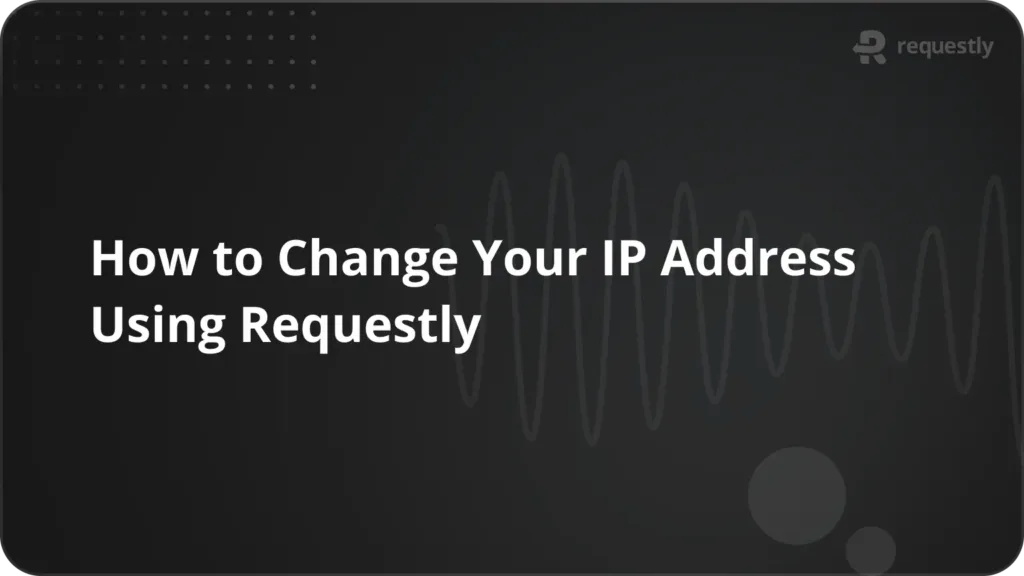
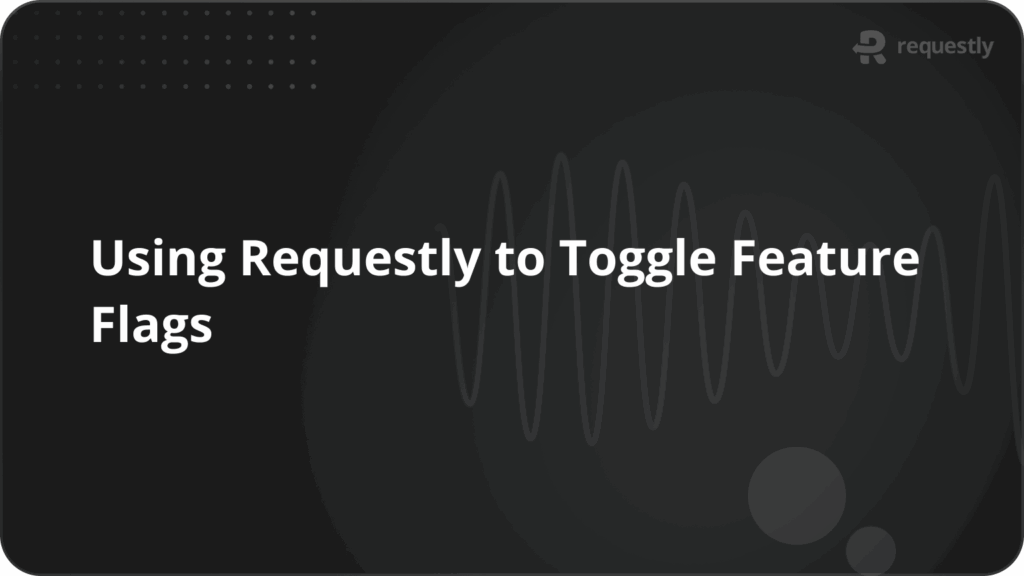
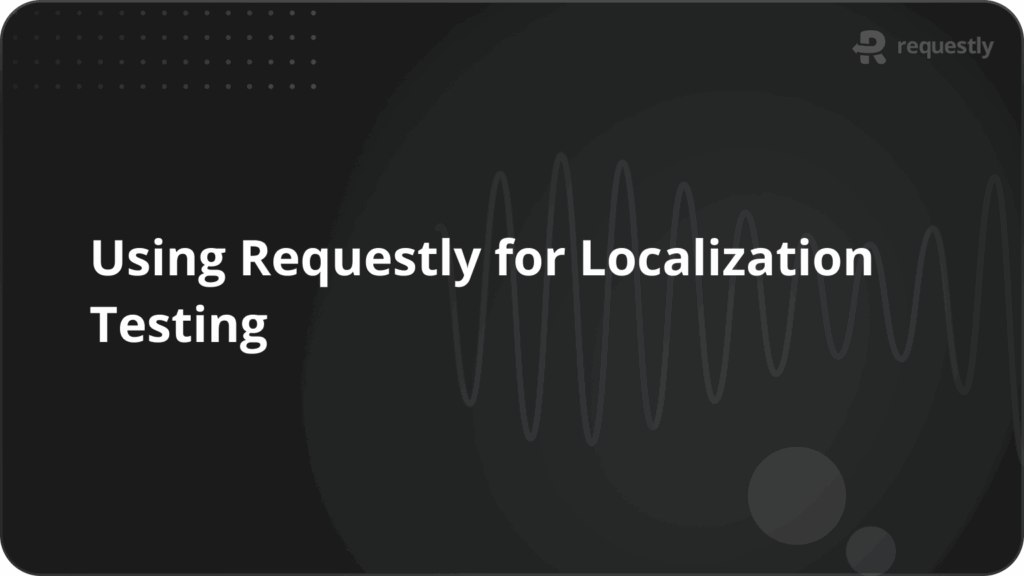