Top interview questions for frontend developer
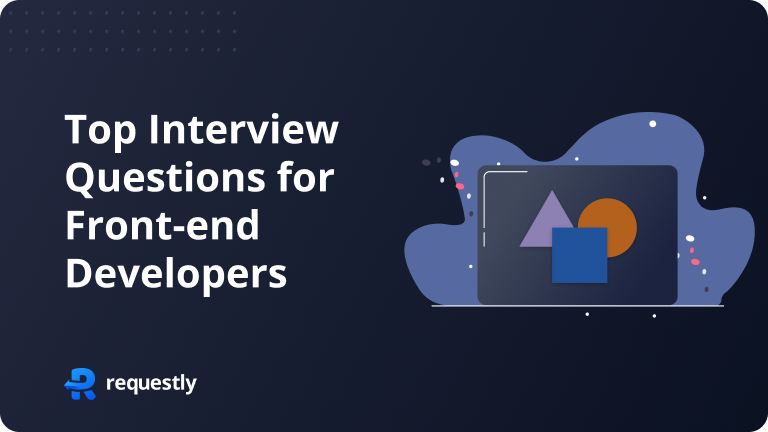
Landing a frontend developer job can be tough due to many technologies and competition. This guide will equip you with the core knowledge to ace those interviews. We’ll break down essential front-end interview questions, covering HTML, CSS, and JavaScript. The questions are grouped based on difficulty level representing the experience level.
Junior Level - Frontend Developer
1. What is the purpose of using the <iframe> tag?
The <iframe>
tag allows you to embed an HTML document within another HTML document, creating a sort of “window” to display external content like videos, maps, or even entire web pages directly on your site. It’s useful for integrating content from different sources seamlessly into your own website. Like youtube videos, google maps can be embedded on any webpage.
2. What is the purpose of using the ‘role’ attribute in HTML?
The role
attribute defines an element’s purpose in the context of accessibility and user interaction. It enhances the semantic meaning of HTML elements for assistive technologies like screen readers, providing them with additional information to understand and interpret content accurately.
For instance, a <div>
element with role="button"
is interpreted as a button by assistive technologies, even though it might not have the default styling or behavior associated with a traditional <button>
element.
Beyond accessibility, role
attributes can also be used to improve interactions with other technologies or tools that parse HTML content.
3. What is responsive design?
Responsive design is an approach to web development that ensures websites adapt gracefully to different screen sizes and devices, providing an optimal viewing and interaction experience for users across desktops, tablets, and mobile phones.
Key components of responsive design include:
- Flexible Grids: Layouts that use relative units (percentages, viewport units) rather than fixed pixels, allowing elements to resize proportionally based on the screen width.
- Fluid Images: Images that adjust their width dynamically to fit their container, preventing them from overflowing on smaller screens.
- Media Queries: CSS rules that apply different styles based on the screen size, allowing for customized layouts, font sizes, and content adjustments for different devices.
Benefits of Responsive Design:
- Improved User Experience: Websites are easier to read and navigate on various devices, leading to increased engagement and conversions.
- SEO Benefits: Google favors mobile-friendly websites in search results, leading to better visibility and organic traffic.
- Cost-Effectiveness: Maintaining a single responsive website is often more efficient than creating separate versions for different devices.
4. What is localStorage?
localStorage
is a Web Storage API provided by browsers that allows you to store key-value pairs of data directly in the user’s browser. This data persists even after the browser is closed and remains available across different browser sessions.
Key characteristics:
- Storage limit: Usually around 5MB per origin (domain).
- Data persistence: Data remains until explicitly cleared by JavaScript code, the user, or the browser itself.
- Scope: Limited to the same origin (protocol, domain, and port).
- API: Simple
setItem
,getItem
,removeItem
,clear
methods for managing data. - Security: Not secure for sensitive data as it can be accessed by any code on the same origin.
Common Use Cases:
- User preferences: Storing theme preferences, language settings, etc.
- Caching data: Storing API responses or other data to reduce network requests.
- Simple state management: Preserving form data, shopping cart contents, etc.
5. What is sessionStorage?
sessionStorage
, like localStorage
, is part of the Web Storage API and allows you to store key-value pairs of data in the user’s browser. However, the key difference lies in its persistence:
- Data lifespan:
sessionStorage
data is stored for the duration of a single browser session (tab). When the tab is closed, the data is cleared. - Scope: Similar to
localStorage
, it’s limited to the same origin.
Use Cases:
- Temporary data: Storing data that’s only needed for the current interaction, like form input values or temporary UI state.
- Sensitive data: Since it’s cleared automatically,
sessionStorage
can be used for slightly more sensitive information thanlocalStorage
, though it’s still not suitable for highly confidential data. - Session-specific settings: Maintaining settings or preferences that should only apply within the current tab or session.
6. What is the use of “use strict” directive in JavaScript?
The "use strict"
directive is a way to voluntarily enforce stricter parsing and error handling on your JavaScript code at runtime. It helps you write cleaner, safer code by preventing certain “sloppy” practices and throwing errors in situations that might otherwise go unnoticed.
Benefits of using "use strict"
:
- Catches common coding mistakes: It throws errors for things like using undeclared variables, accidentally assigning values to read-only properties, or using reserved keywords as variable names.
- Prevents accidental globals: In non-strict mode, assigning a value to an undeclared variable creates a global variable. In strict mode, this throws an error, forcing you to explicitly declare your variables.
- Eliminates “silent errors”: Some JavaScript errors don’t throw exceptions by default (e.g., assigning a value to a non-writable property). Strict mode makes these errors visible, making it easier to debug.
- Restricts unsafe features: It disallows certain features considered problematic or potentially unsafe, such as the
with
statement and the use ofarguments.callee
.
How to use it:
- Global: Place
"use strict";
at the beginning of a script file to enable strict mode for the entire file. - Function-level: Place
"use strict";
at the beginning of a function body to enable it only within that function.
Important:
- Strict mode is enabled by default in ES modules and in classes.
- It’s a good practice to use
"use strict"
in modern JavaScript development to improve code quality and catch potential issues early on.
7. Difference between null and undefined in JS.
In JavaScript, both null
and undefined
represent the absence of a meaningful value, but they differ in their semantics and use cases:
Features | undefined | null |
---|---|---|
Type | undefined (primitive type) | object (but behaves like a primitive value) |
Meaning | Variable declared but not assigned a value. | Intentional absence of an object value. |
Assignment | Implicitly assigned by JavaScript. | Explicitly assigned by the developer. |
Comparison | Loosely equal to null ( undefined == null ) | Loosely equal to undefined |
Typeof | typeof undefined returns “undefined” | typeof null returns “object” |
Use Cases | Typically used to indicate that a variable has been declared but hasn’t been initialized or assigned a value yet. It can also be the result of accessing a non-existent property of an object. | Generally used to explicitly indicate the absence of an object value. It’s often used to represent the intentional absence of data or to clear the value of a variable that previously held an object reference. |
8. What is callback hell and how to avoid it?
Callback hell, also known as the “pyramid of doom,” is a situation in JavaScript where you have multiple nested callbacks, leading to code that’s difficult to read, understand, and maintain. It typically arises when dealing with asynchronous operations, where each operation depends on the completion of the previous one.
How to avoid callback hell:
- Promises: Promises provide a cleaner way to handle asynchronous operations by allowing you to chain
.then()
handlers for success and.catch()
for errors. - Async/await: Introduced in ES2017,
async/await
makes asynchronous code look and behave more like synchronous code, improving readability and maintainability. - Modularization: Break down complex logic into smaller, reusable functions or modules, making it easier to manage and test individual parts.
- Libraries/Frameworks: Utilize libraries or frameworks that provide higher-level abstractions for handling asynchronous operations, such as RxJS or Redux Saga.
9. Explain debouncing in JavaScript?
Debouncing is a technique in JavaScript that limits the rate at which a function can be executed. It works by delaying the execution of a function until a certain amount of time has passed since the last trigger event.
Why use debouncing?
- Improves performance: Prevents functions from being called excessively, which can lead to performance issues, especially for events that trigger rapidly (like scrolling or resizing).
- Optimizes resource usage: Reduces unnecessary calculations or network requests.
- Provides a better user experience: Makes interactions smoother and less disruptive.
Common use cases:
- Search suggestions: Delay fetching suggestions until the user pauses typing.
- Auto-saving forms: Save data only after the user stops making changes.
- Resizing elements: Recalculate layout only after the resizing action is complete.
function debounce(func, delay) {
let timeoutId;
return function(...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
const handleResize = debounce(() => {
// Code to execute after resizing is complete
}, 250); // Delay of 250 milliseconds
window.addEventListener('resize', handleResize);
10. Explain the concept of currying in JavaScript?
Currying is a technique in functional programming where a function that takes multiple arguments is transformed into a sequence of functions, each taking a single argument. In other words, it breaks down a multi-argument function into a series of nested functions, where each function takes one argument and returns another function that takes the next argument, and so on, until all arguments are consumed.
function add(x) {
return function(y) {
return function(z) {
return x + y + z;
};
};
}
// Curried call
const result = add(2)(3)(4); // Output: 9
// Partially applied functions
const add2 = add(2);
const add2and3 = add2(3);
const result2 = add2and3(4); // Output: 9
Benefits of currying:
- Partial Application: You can create partially applied functions by providing some arguments upfront and then using them later with the remaining arguments.
- Code Reusability: Curried functions are more modular and composable, allowing you to reuse them in different parts of your code.
- Readability: The code can be more readable and expressive, especially when dealing with functions that take many arguments.
- Function Composition: Curried functions can be easily composed together to create new functions, which is a powerful technique in functional programming.
11. Describe the concept of CORS.
Cross-Origin Resource Sharing (CORS) is a browser-based security mechanism that allows a web page from one domain to access resources from a different domain. It’s a relaxation of the Same-Origin Policy, which typically restricts such interactions for security reasons.
How CORS Works:
- Request: A browser sends a request to a server on a different domain (cross-origin request).
- Headers: The server responds with specific HTTP headers that indicate whether the requesting origin is allowed to access the resource.
- Browser Check: The browser examines the headers to determine if the cross-origin request is permitted based on the provided CORS configuration.
- Access Granted/Denied: If allowed, the browser processes the response; otherwise, it blocks the request.
Key CORS Headers:
Access-Control-Allow-Origin
: Specifies which origins are allowed to access the resource (e.g., “ for all, or a specific domain).Access-Control-Allow-Methods
: Indicates the allowed HTTP methods (e.g., GET, POST, PUT).Access-Control-Allow-Headers
: Lists the allowed request headers.Access-Control-Max-Age
: Caches the results of a preflight request for a specified time.
Access-Control-Allow-Origin: https://example.com
Access-Control-Allow-Methods: GET, POST
Preflight Requests:
For requests that could potentially modify data (e.g., PUT, DELETE), the browser might send an OPTIONS request first to check if the actual request is allowed, based on the CORS headers.
Why CORS is Important:
- Enables building web applications that consume APIs or resources from different domains.
- Facilitates secure cross-origin communication while protecting against unauthorized access.
- Crucial for Single Page Applications (SPAs) and modern web development practices.
Senior Level
1. What is difference between visibility: hidden
and display: none
properties in CSS?
Both visibility: hidden
and display: none
hide elements visually, but with key differences:
visibility: hidden
- Hides the element, but it still occupies space in the layout.
- The element is not visible, but its position and dimensions are maintained.
- Events (like click) associated with the hidden element are not triggered.
display: none
- Completely removes the element from the layout flow.
- It behaves as if the element was not present in the document.
- The element does not occupy space, and its position is filled by other elements.
- Events associated with the element are not triggered.
2. What is the benefit of srcset
?
The srcset
attribute allows you to provide multiple versions of an image at different resolutions or sizes. This enables the browser to choose the most appropriate image based on the user’s device characteristics (screen size, pixel density, etc.) and network conditions.
Key benefits of srcset
:
- Performance: Prevents loading unnecessarily large images on smaller devices, improving load times and saving bandwidth.
- Responsiveness: Ensures your images look sharp on high-resolution displays while maintaining reasonable sizes on lower-resolution devices.
- Art Direction: Can be used with the
<picture>
element to serve entirely different images based on specific breakpoints, allowing for optimized compositions or cropped versions for different screen sizes.
<img srcset="image-small.jpg 480w, image-medium.jpg 800w, image-large.jpg 1200w"
sizes="(max-width: 600px) 480px, (max-width: 900px) 800px, 1200px"
src="image-medium.jpg"
alt="A responsive image">
3. What is User Centered Design?
User-Centered Design (UCD) is an iterative design approach that prioritizes the needs, preferences, and behaviors of the end-users throughout the entire design and development process.
It involves:
- Understanding Users: Conducting user research to gain insights into their goals, motivations, pain points, and mental models.
- Defining Requirements: Clearly articulating the user’s needs and expectations as design requirements.
- Designing Solutions: Creating design concepts and prototypes that address the user’s needs and are easy to use.
- Evaluating with Users: Testing designs with real users to gather feedback and identify areas for improvement.
- Iterating: Continuously refining designs based on user feedback and insights until they meet the desired level of usability and satisfaction.
UCD aims to create products and services that are intuitive, efficient, and enjoyable to use, ultimately leading to increased user satisfaction, engagement, and loyalty.
4. What is Mixin?
In frontend development, a mixin is a reusable block of code that can be included in other parts of your project, typically within CSS preprocessors (like Sass or Less) or JavaScript frameworks (like Vue or React).
Think of it as a template for styles or functionality that can be easily shared and customized in different contexts.
CSS Mixins:
- Contain reusable CSS properties or declarations.
- Can be parameterized to allow for customization.
- Reduce code duplication and improve maintainability.
@mixin button-styles($background-color, $text-color) {
background-color: $background-color;
color: $text-color;
padding: 10px 20px;
}
.primary-button {
@include button-styles(blue, white);
}
.secondary-button {
@include button-styles(gray, black);
}
JavaScript Mixins:
- Offer reusable methods or properties.
- Can be “mixed into” components or classes.
- Promote modularity and code organization.
const myMixin = {
methods: {
showAlert() {
alert('Hello from the mixin!');
}
}
};
new Vue({
mixins: [myMixin],
// ...
});
5. What is the purpose of passing defer or async attributes to the script tag?
The defer
and async
attributes control how external scripts are loaded and executed within an HTML document. In both cases scripts are downloaded in parallel with HTML parsing.
The only difference lies in the execution order. In case of defer
scripts are executed in the order they appear in the document only after HTML has been fully parsed. While in case of async
scripts start executing as soon as they finish downloading. By default scripts are loaded and executed synchronously, blocking the rendering of subsequent page content until they are finished.
We use defer for scripts that need a fully parsed DOM while async can be used where DOM parsing is not required like a chat widget.
6. What are CSS preprocessors and their advantage over pure CSS?
CSS preprocessors are scripting languages that extend the capabilities of regular CSS, allowing you to write more organized, efficient, and maintainable stylesheets. Popular examples include Sass, Less, and Stylus.
Advantages over pure CSS:
- Variables: Store reusable values like colors, fonts, or spacing, making it easier to maintain consistency and update styles across your project.
- Nesting: Write selectors in a nested format, mirroring the structure of your HTML, resulting in cleaner and more readable code.
- Mixins: Create reusable blocks of styles that can be included in multiple selectors, reducing repetition and promoting DRY (Don’t Repeat Yourself) principles.
- Functions: Perform calculations, manipulate colors, or generate repetitive patterns, adding a layer of logic and flexibility to your stylesheets.
- Inheritance: Extend styles from one selector to another, simplifying the creation of complex styles and reducing code duplication.
- Importing: Split your stylesheets into smaller, more manageable files and then combine them into a single CSS file for production.
7. What is event propagation in JavaScript?
Event propagation in JavaScript refers to how events are triggered and travel through the Document Object Model (DOM) tree when an element is interacted with. It determines the order in which event handlers attached to different elements are executed.
There are two main phases of event propagation:
- Capturing Phase: The event starts at the root of the DOM (the
window
object) and travels down through each ancestor element towards the target element that triggered the event. - Bubbling Phase: The event “bubbles” up from the target element, going through each ancestor element until it reaches the root.
Key Points:
- By default, event handlers are registered for the bubbling phase.
- You can register handlers for the capturing phase by passing
true
as the third argument toaddEventListener
. - You can stop event propagation using
event.stopPropagation()
. - Event propagation is useful for creating more efficient event handling systems and managing events at higher levels in the DOM hierarchy.
8. What is event delegation in JavaScript?
Event delegation is a technique in JavaScript where you add a single event listener to a parent element instead of adding individual event listeners to each of its child elements. When an event occurs on any of the child elements, it “bubbles up” through the DOM tree to the parent element, where the event handler is triggered.
How it works:
- Event Listener: You attach an event listener (e.g.,
click
) to a parent element that contains multiple child elements. - Event Bubbling: When an event (like a click) occurs on a child element, it travels up through the DOM hierarchy to its parent element.
- Event Handling: The event handler on the parent element checks the
event.target
property to identify the specific child element that was clicked. - Conditional Logic: You use conditional logic (often based on
event.target.tagName
or other attributes) to determine how to handle the event for that specific child element.
<ul id="my-list">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<script>
document.getElementById('my-list').addEventListener('click', (event) => {
if (event.target.tagName === 'LI') {
console.log('Clicked on:', event.target.textContent);
}
});
</script>
Advantages of Event Delegation:
- Efficiency: Fewer event listeners are needed, which can improve performance, especially with large numbers of elements.
- Dynamic Elements: Works seamlessly with elements added or removed dynamically from the DOM, as the parent’s event listener automatically handles new elements.
- Cleaner Code: Reduces code redundancy and improves maintainability.
Common Use Cases:
- Handling events on list items, table rows, or other dynamically generated content.
- Creating custom interactive elements (e.g., image galleries, tooltips) where you don’t know the exact number of child elements in advance.
9. What is event bubbling in JavaScript?
Event bubbling is a mechanism in JavaScript where events triggered on a nested element (child) also trigger on its parent element(s), propagating up the Document Object Model (DOM) tree until it reaches the root element (window).
How it works:
- Event Occurs: An event like a click happens on the innermost element (the button).
- Propagation: The event then “bubbles” up, triggering handlers on its ancestors in order, until it reaches the outermost container or the
window
object.
Use Cases:
- Event Delegation: Attaching a single event handler to a parent element to manage events on multiple child elements, improving performance and simplifying code.
- Custom Events: Creating custom events that trigger on a child element and are listened to by parent elements, allowing for better component communication.
10. What’s the difference between debouncing and throttling?
Debouncing and throttling are both techniques used to control the rate at which a function is executed, but they work in slightly different ways and are best suited for different scenarios.
Debouncing:
- Purpose: Delays the execution of a function until a certain amount of time has passed since the last trigger event.
- How it works: When an event occurs, a timer is set. If the event occurs again before the timer expires, the timer is reset. The function is executed only when the timer finally expires without being reset.
- Use cases:
- Search input suggestions: Wait for the user to finish typing before fetching suggestions.
- Button click handlers: Prevent multiple clicks from triggering the same action.
- Window resize events: Recalculate layout only after the user finishes resizing.
Throttling:
- Purpose: Limits the maximum rate at which a function is executed, allowing it to run at regular intervals.
- How it works: The function is executed immediately the first time it’s triggered, then a timer is set. Subsequent triggers are ignored until the timer expires, at which point the function is executed again and the timer is reset.
- Use cases:
- Infinite scrolling: Load new content only at specific intervals while the user scrolls.
- Mouse movement tracking: Update cursor position or perform other actions at a controlled rate.
- Game animation loops: Maintain a consistent frame rate.
11. What is Transducer?
In JavaScript, a transducer is a function that transforms a reducer. It’s a higher-order function that takes one reducer and returns another reducer with modified behavior. Transducers are designed to improve the efficiency and flexibility of data processing pipelines.
How Transducers Work:
- Reducer: A reducer is a function that takes an accumulator and a value, and returns a new accumulator. Reducers are used to combine values from a collection into a single result.
- Transducer: A transducer is a function that takes a reducer as input and returns a new reducer. This new reducer will apply a transformation to the values before passing them to the original reducer.
- Composition: Transducers can be composed together using function composition (
compose()
), allowing you to build complex data processing pipelines from simple transformations.
Benefits of Transducers:
- Efficiency: Transducers are often more efficient than traditional array methods like
map
,filter
, andreduce
when you have multiple transformations to apply to a collection. This is because transducers can process data in a single pass, avoiding the creation of intermediate arrays. - Flexibility: Transducers are composable, which means you can easily create new transformations by combining existing ones. This makes it easier to create complex data processing pipelines.
- Lazy Evaluation: Transducers can be used for lazy evaluation, meaning that they only process values as they are needed. This can be useful when dealing with large datasets or infinite sequences.
const map = (fn) => (reducer) => (acc, val) => reducer(acc, fn(val));
const filter = (predicate) => (reducer) => (acc, val) => predicate(val) ? reducer(acc, val) : acc;
const data = [1, 2, 3, 4];
const result = data.reduce(compose(
map(x => x * 2),
filter(x => x % 2 === 0)
), 0); // Output: 12
12. What is Content Security Policy (CSP)?
Content Security Policy (CSP) is a security mechanism that helps protect websites from attacks like cross-site scripting (XSS), clickjacking, and other code injection vulnerabilities. It works by defining a whitelist of trusted sources of content that the browser is allowed to load for a given page.
Key Points:
- Header-based: CSP is typically implemented as an HTTP response header sent by the server.
- Directives: CSP uses directives to specify allowed sources for different types of content (e.g.,
script-src
,style-src
,img-src
, etc.). - Default Deny: By default, CSP blocks anything that isn’t explicitly allowed by the policy.
- Flexibility: CSP allows you to fine-tune the policy to match your specific security requirements.
- Reporting: It can also send reports about policy violations to a specified endpoint for monitoring and analysis.
Benefits:
- Defense-in-Depth: Provides an extra layer of security on top of other measures.
- Prevention: Helps prevent malicious scripts from executing on your website.
- Monitoring: Allows you to detect and investigate potential attacks.
- Backward Compatibility: Works with most modern browsers.
Considerations:
- Complexity: CSP can be complex to configure and maintain, especially for larger websites.
- False Positives: Incorrectly configured policies can block legitimate content.
13. What is Cross-Site Scripting (XSS)?
Cross-Site Scripting (XSS) is a security vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. It occurs when a web application includes untrusted user-supplied data without proper validation or sanitization, allowing attackers to execute arbitrary JavaScript code in the victim’s browser.
Types of XSS:
- Reflected XSS: The malicious script is part of the victim’s request and is reflected back in the server’s response. It often occurs through manipulating URLs or form submissions.
- Stored XSS: The script is stored on the server-side (e.g., in a database) and is executed every time a user loads the affected page. This can have a broader impact as it affects multiple users.
- DOM-based XSS: The vulnerability exists in the client-side code due to how the page processes and modifies its DOM based on user input.
Impact of XSS:
- Data Theft: Attackers can steal sensitive information like cookies, session tokens, or user credentials.
- Account Takeover: XSS can be used to impersonate the victim and perform actions on their behalf.
- Malicious Redirects: Users can be redirected to phishing or malware-laden sites.
- Defacement of Websites: Attackers can alter the appearance or content of a website.
Prevention:
- Input Validation: Always validate and sanitize user input before using it in your application.
- Output Encoding: Encode user input when displaying it in HTML, JavaScript, or other contexts.
- Content Security Policy (CSP): A security mechanism that defines trusted sources of content, helping prevent XSS attacks.
- HttpOnly Cookies: Set cookies with the HttpOnly flag to prevent them from being accessed by client-side scripts.
14. What is ClickJacking?
Clickjacking, also known as a “UI redress attack”, is a malicious technique where an attacker tricks a user into clicking on something different from what they perceive. This is achieved by layering invisible or disguised elements on top of a legitimate website, leading the user to unintentionally perform actions they didn’t intend.
How it works:
- Hidden Overlay: Attackers embed a transparent iframe or other element over the targeted website.
- Deceptive UI: This overlay contains disguised buttons, links, or other interactive elements positioned over legitimate ones.
- Misleading Interaction: When a user clicks on what they believe to be a harmless element, they are actually clicking on the hidden malicious element, triggering unintended actions like:
- Changing settings
- Making purchases
- Sharing personal information
- Downloading malware
Example:
An attacker might place an invisible “Like” button over a video play button on a social media site. When a user clicks to play the video, they unknowingly “Like” a page or post they didn’t intend to.
Prevention:
- X-Frame-Options header: A server-side response header that instructs the browser whether or not a page can be displayed within an iframe.
- Content Security Policy (CSP): Defines a policy for the browser, specifying the sources from which content can be loaded, helping mitigate clickjacking attempts.
- Frame-Busting Scripts: Client-side JavaScript code that detects if a page is loaded within an iframe and attempts to break out of it.
15. What does SOLID stand for? How do you use?
SOLID is an acronym for five design principles in object-oriented programming that, when followed together, make software designs more understandable, flexible, and maintainable:
S – Single Responsibility Principle (SRP): A class should have only one reason to change. In other words, it should have only one job or responsibility.
O – Open/Closed Principle (OCP): Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means you should be able to add new functionality without changing existing code.
L – Liskov Substitution Principle (LSP): Objects in a program should bereplaceable with instances of their subtypes without altering the correctness of the program. In simpler terms, derived classes should be able to substitute their base classes without causing unexpected behavior.
I – Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they do not use. It’s better to have many smaller, client-specific interfaces than one general-purpose interface.
D – Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules; both should depend on abstractions. Abstractions should not depend on details; details should depend on abstractions.
How I use SOLID principles:
- SRP: I try to keep my classes and functions focused on a single task. When a class starts doing too much, I look for ways to break it down into smaller, more cohesive units.
- OCP: I design my code to be extensible by using interfaces, abstract classes, and composition. This allows me to add new features without modifying existing code, reducing the risk of introducing bugs.
- LSP: I make sure that derived classes can be used interchangeably with their base classes. This ensures that my code is predictable and reliable.
- ISP: I avoid creating large, bloated interfaces. Instead, I define smaller, more specific interfaces that are tailored to the needs of the clients that use them.
- DIP: I depend on abstractions rather than concrete implementations. This makes my code more flexible and easier to test. I can easily swap out different implementations of an abstraction without affecting the rest of my code.
16. What is meant by the KISS principle?
The KISS principle, often expanded as “Keep It Simple, Stupid,” is a design principle that advocates for simplicity in software development and engineering. It emphasizes that most systems work best when they are kept simple rather than made complex. Therefore, simplicity should be a key goal in design, and unnecessary complexity should be avoided.
In frontend development, KISS means:
- Writing Clean and Readable Code: Avoiding overly complex structures, deeply nested functions, or confusing syntax.
- Favor Straightforward Solutions: Choosing simpler solutions over complicated ones, even if the latter seem more “clever” or “elegant.”
- Breaking Down Complex Tasks: Decomposing large problems into smaller, more manageable components.
- Prioritizing User Experience: Focusing on creating intuitive interfaces and interactions that users can easily understand and use.
Contents
- Junior Level - Frontend Developer
- 1. What is the purpose of using the <iframe> tag?
- 2. What is the purpose of using the ‘role’ attribute in HTML?
- 3. What is responsive design?
- 4. What is localStorage?
- 5. What is sessionStorage?
- 6. What is the use of “use strict” directive in JavaScript?
- 7. Difference between null and undefined in JS.
- 8. What is callback hell and how to avoid it?
- 9. Explain debouncing in JavaScript?
- 10. Explain the concept of currying in JavaScript?
- 11. Describe the concept of CORS.
- Senior Level
- 1. What is difference between visibility: hidden and display: none properties in CSS?
- 2. What is the benefit of srcset?
- 3. What is User Centered Design?
- 4. What is Mixin?
- 5. What is the purpose of passing defer or async attributes to the script tag?
- 6. What are CSS preprocessors and their advantage over pure CSS?
- 7. What is event propagation in JavaScript?
- 8. What is event delegation in JavaScript?
- 9. What is event bubbling in JavaScript?
- 10. What’s the difference between debouncing and throttling?
- 11. What is Transducer?
- 12. What is Content Security Policy (CSP)?
- 13. What is Cross-Site Scripting (XSS)?
- 14. What is ClickJacking?
- 15. What does SOLID stand for? How do you use?
- 16. What is meant by the KISS principle?
Subscribe for latest updates
Share this article
Related posts
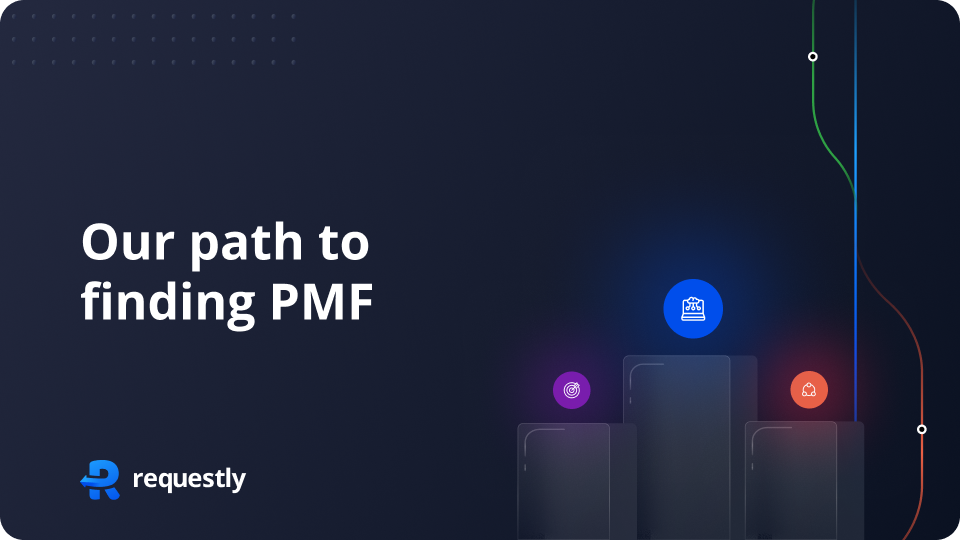
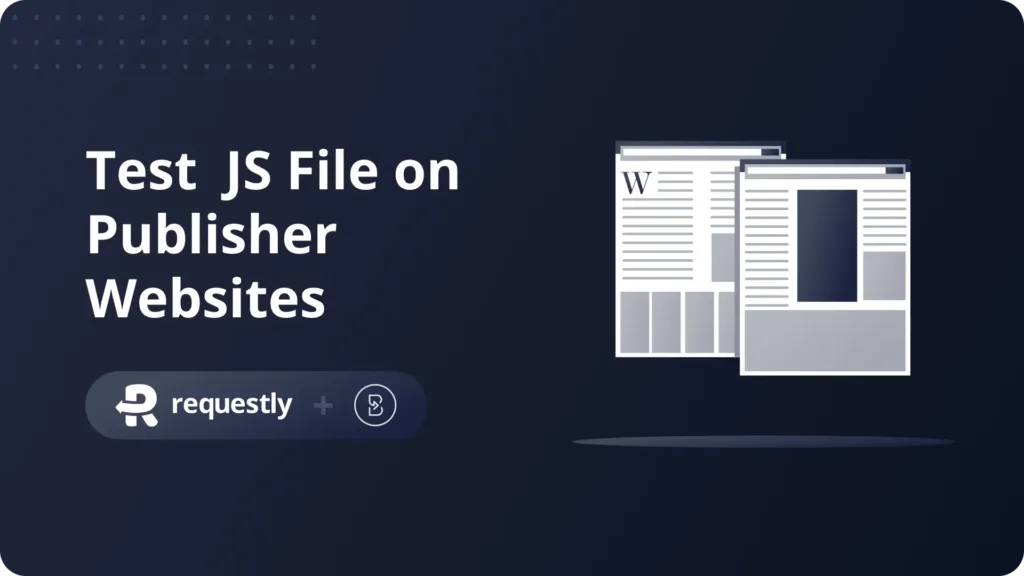
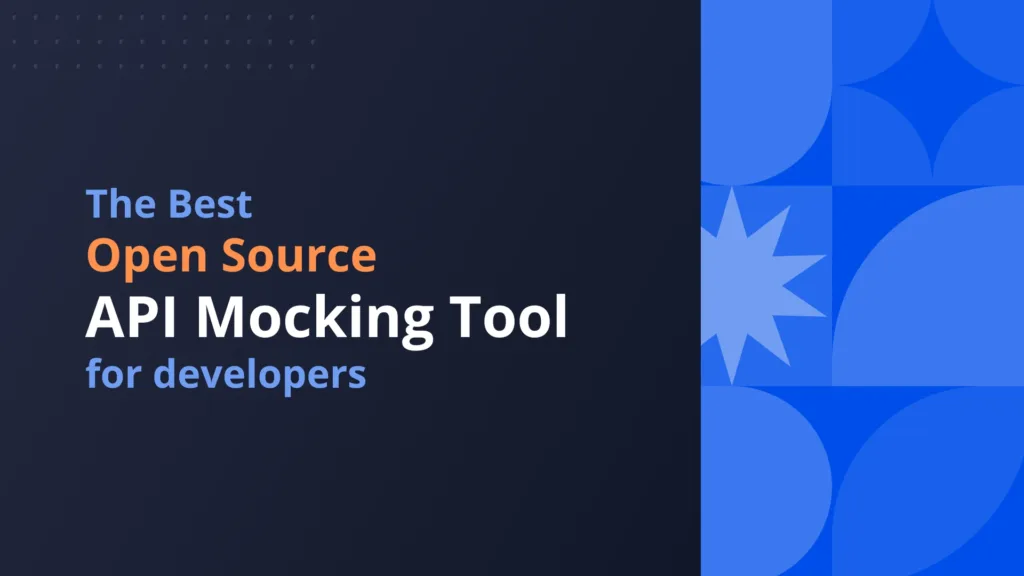