What is HTTP Interceptor & Why Should You Use One?
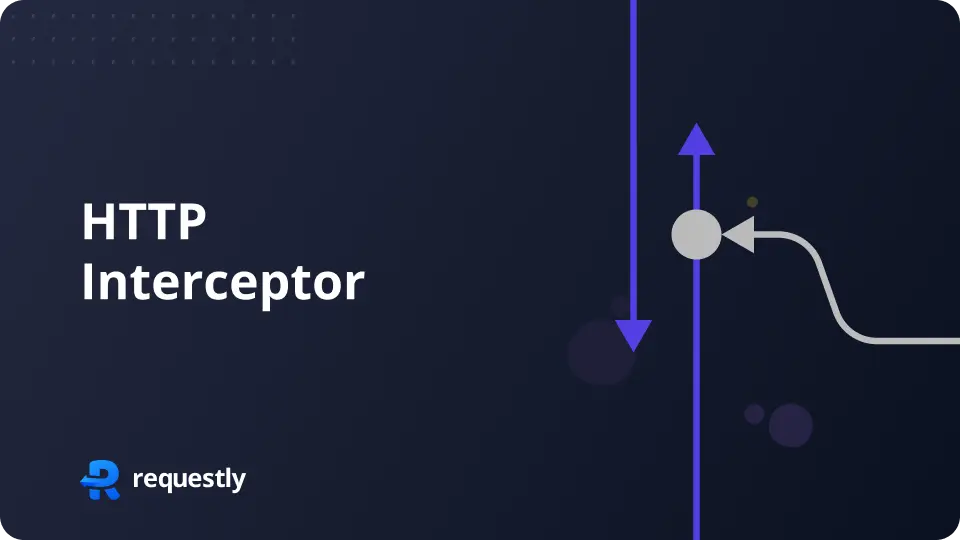
Introduction
When building web applications, ensuring smooth communication between the client and the server is important. HTTP interceptor help streamline this communication. Using HTTP interceptors, you can catch and modify HTTP requests and responses before they reach your application. You can control your requests by adding authentication tokens, logging, dealing with errors, and changing data all in one place. In this blog, we’ll look at what HTTP interceptors are, how they work, and how they can make your web development life simpler and more efficient. Whether you’re developing with Angular, React, Android, iOS, Axios, or another HTTP library, understanding interceptors will give you more control over your app’s communication with the server.
Understanding HTTP Interceptor
HTTP interceptors operate as intermediaries between your application and the server, playing a pivotal role in the lifecycle of HTTP requests and responses. When an application makes an HTTP call, the request first passes through the interceptor, where it can be examined, modified, or logged before being sent to the server. Similarly, when the server responds, the response can be intercepted, processed, or transformed before it reaches the application.
The true power of HTTP interceptors lies in their ability to centralize and simplify HTTP handling within your app. Instead of duplicating code across various components to handle tasks like authentication, error handling, or logging, you can define these behaviors in one place. This global handling approach not only reduces redundancy but also makes the application easier to maintain and scale.
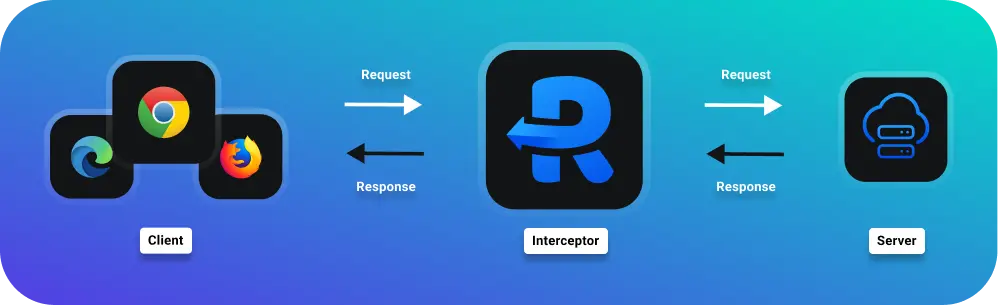
For instance, consider a scenario where you need to automatically attach a language preference header to every HTTP request based on the user’s settings. Instead of adding this header manually in every service or component that makes HTTP calls, you can configure an interceptor to append the language header to all outgoing requests. This ensures a consistent implementation and significantly reduces the potential for errors or omissions.
Additionally, interceptors can be used for performance monitoring, request caching, and transforming response data, making them indispensable tools in modern web development.
By understanding how to effectively implement and utilize HTTP interceptors, developers can enhance the efficiency, security, and reliability of their applications, leading to a smoother and more streamlined development process.
Benefits of Using HTTP Interceptors
Using HTTP interceptors in web development offers several significant benefits that enhance both the development process and the performance of applications:
- Centralized Logic: HTTP interceptors allow developers to centralize common functionalities such as authentication, error handling, logging, and header management. Instead of implementing these features across multiple endpoints or services, interceptors enable you to handle them in a single location. This approach reduces redundancy, improves code maintainability, and ensures consistency across your application.
- Code Reusability: By encapsulating HTTP logic within interceptors, developers can reuse these interceptors across different parts of the application. This reusability not only saves development time but also minimizes the risk of introducing bugs or inconsistencies when implementing similar functionalities in multiple places.
- Global Effect: Interceptors operate globally within an application, intercepting every HTTP request and response. This global scope allows you to enforce application-wide policies and behaviors uniformly. For instance, you can enforce authorization checks or add headers to outgoing requests without modifying individual components, thereby promoting a more systematic approach to application development.
- Performance Optimization: HTTP interceptors can be used to optimize performance by implementing caching mechanisms, compressing request payloads, or handling response caching. These optimizations help reduce network overhead and improve application responsiveness, leading to a better user experience.
- Security Enhancements: Implementing security measures such as CSRF token validation, CORS handling, or SSL configuration can be efficiently managed through HTTP interceptors. By integrating these security checks at the interceptor level, you ensure consistent and robust security practices across your application.
- Debugging and Logging: Interceptors are invaluable for debugging purposes as they allow developers to log HTTP requests and responses, monitor network traffic, and inspect data transformations. This visibility aids in diagnosing issues, tracking performance metrics, and ensuring the reliability of your application.
- Scalability and Flexibility: As applications evolve, interceptors provide flexibility to adapt and extend functionality without extensive refactoring. Whether you need to introduce new features, update existing policies, or integrate with external services, interceptors offer a flexible mechanism to accommodate these changes seamlessly.
Use Cases for HTTP Interceptors
HTTP interceptors offer versatile solutions to various common challenges in web development, providing developers with powerful tools to enhance functionality, security, and performance across applications. Here are several compelling use cases where HTTP interceptors prove invaluable:
- Authentication and Authorization : Implementing authentication mechanisms like adding tokens, validating user sessions, or enforcing access controls can be efficiently managed through HTTP interceptors. By intercepting outgoing requests, developers can automatically append authentication headers or verify user credentials without modifying individual API calls.
- Toggling staging environment : Switching between staging, local and production environments using HTTP interceptors allows developers to dynamically adjust URLs and request parameters. This flexibility allows for easy testing across different environments without altering core application code.
- Request and Response Transformation : Interceptors enable developers to preprocess request payloads or manipulate response data before they reach the application. This capability is particularly useful for converting data formats, compressing responses, or transforming API responses into a standardized format that aligns with application requirements.
- Performance Optimization : By leveraging interceptors, developers can implement caching strategies to store frequently accessed data locally or handle response caching to reduce server load and enhance application responsiveness. Interceptors can also optimize network traffic by compressing request payloads or managing resource-intensive operations efficiently.
- Monitoring and Analytics : Integrating monitoring and analytics capabilities within HTTP interceptors allows developers to capture metrics such as response times, request frequencies, or API usage patterns. This data can be instrumental in identifying performance bottlenecks, optimizing resource allocation, and making informed decisions to enhance application performance.
Tools and Libraries for HTTP Interception
Several tools and libraries support HTTP interception, each offering unique features and capabilities suited to different frameworks and use cases. Here are some popular options:
Axios
Axios is a popular JavaScript library used to make HTTP requests from the browser or Node.js. It provides a simple API for sending asynchronous HTTP requests to REST endpoints and performing CRUD operations.
How to Use Axios for HTTP Interception:
1. Installation:
npm install axios
2. Creating an Interceptor:
import axios from 'axios';
// Add a request interceptor
axios.interceptors.request.use(function (config) {
// Do something before request is sent
console.log('Request Interceptor', config);
return config;
}, function (error) {
// Do something with request error
return Promise.reject(error);
});
// Add a response interceptor
axios.interceptors.response.use(function (response) {
// Do something with response data
console.log('Response Interceptor', response);
return response;
}, function (error) {
// Do something with response error
return Promise.reject(error);
});
Angular HttpClient
Angular HttpClient is a built-in service in Angular for making HTTP requests. It offers advanced capabilities, including the ability to intercept HTTP requests and responses.
How to Use Angular HttpClient for HTTP Interception:
1. Installation:
ng add @angular/common/http
2. Creating an Interceptor:
// Import necessary Angular core and HTTP client modules
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable()
// Define the interceptor class that implements the HttpInterceptor interface
export class MyInterceptor implements HttpInterceptor {
// Implement the intercept method which will be called for every HTTP request
intercept(req: HttpRequest, next: HttpHandler): Observable> {
// Clone the request and modify it to include the Authorization header with a token
const modifiedReq = req.clone({
headers: req.headers.set('Authorization', `Bearer my-token`)
});
// Pass the modified request to the next handler in the chain
return next.handle(modifiedReq);
}
}
3. Registering the Interceptor:
// Import necessary Angular HTTP client modules and the interceptor
import { HTTP_INTERCEPTORS } from '@angular/common/http';
import { MyInterceptor } from './my-interceptor';
@NgModule({
// Provide the interceptor in the application module
providers: [
// Register the interceptor with the HTTP_INTERCEPTORS injection token
// `multi: true` allows multiple interceptors to be registered
{ provide: HTTP_INTERCEPTORS, useClass: MyInterceptor, multi: true },
],
})
export class AppModule {}
HTTP Toolkit
HTTP Toolkit is a powerful, open-source tool specifically designed to intercept, inspect, and modify HTTP(s) traffic. This versatile tool proves to be extremely useful for a wide range of professionals including developers, testers, and security experts. These individuals often need to analyze network traffic, debug APIs, or thoroughly test web applications to ensure their proper functioning and security. HTTP Toolkit provides an intuitive and user-friendly interface that makes it easy to capture HTTP requests and responses. Furthermore, it can decrypt HTTPS traffic, which is essential for examining encrypted data. The tool also allows users to manipulate this data in real-time, providing immediate feedback and enabling quick adjustments. This capability is particularly beneficial for those conducting detailed analysis or troubleshooting complex issues within their network environments.
How to use HTTP Toolkit:
- Installation: First, download HTTP Toolkit latest version for you operating system. Follow the on-screen instructions to complete the installation process on your computer.
- Capture Traffic: After the installation is complete, you can start instances of the installed web browser. For this demonstration, we will use Google Chrome.
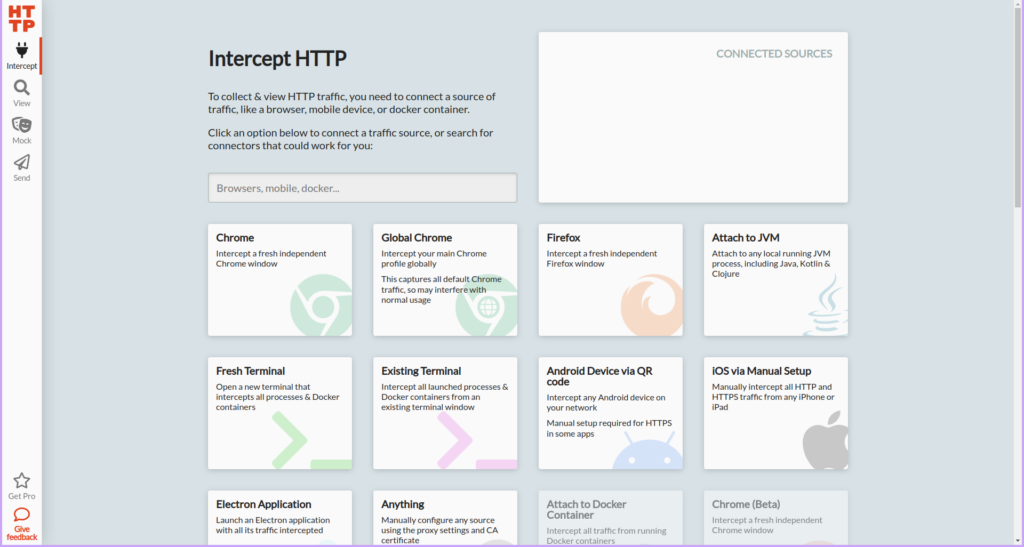
- Once you launch Chrome, you should see a tab opened by HTTP Toolkit. This tab means that http toolkit is active and it will allow you to monitor and capture all HTTP and HTTPS traffic from the browser.
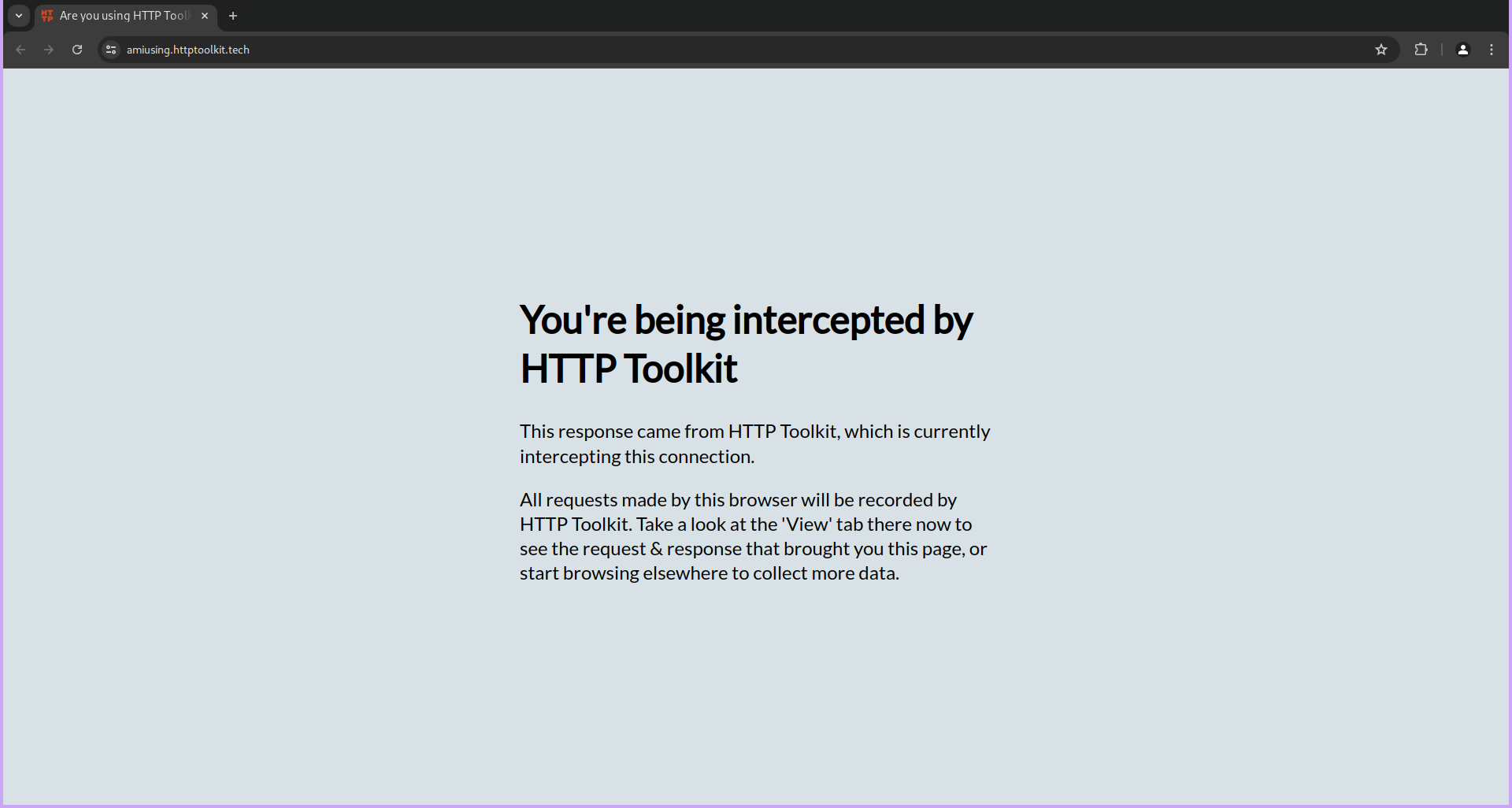
- Now, navigate to any website, for instance, google.com, by typing the URL in the address bar and pressing Enter.
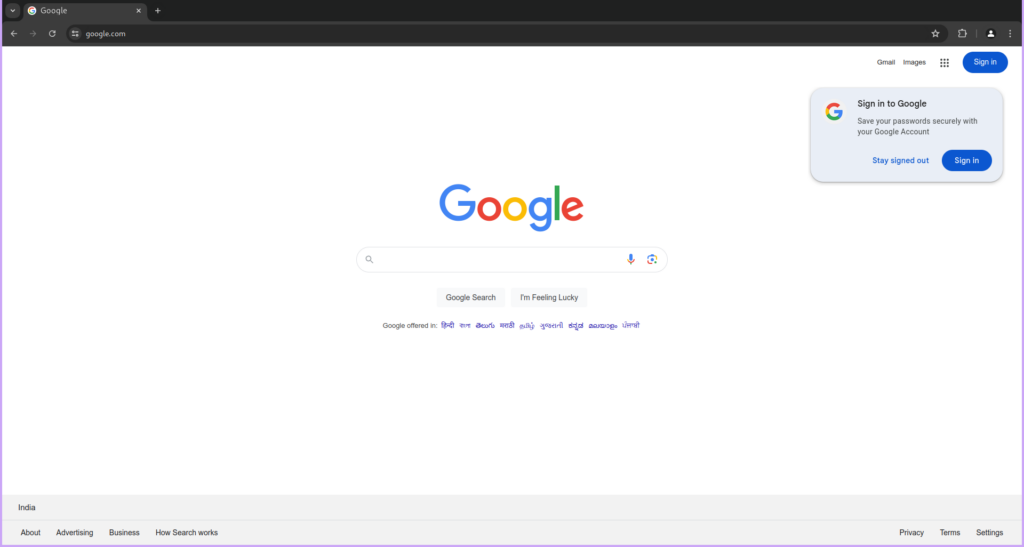
- As the webpage loads, HTTP Toolkit will automatically capture all the requests made to load the various elements of the page, such as HTML, CSS, JavaScript, images, and more. You will be able to see a detailed list of these requests in the HTTP Toolkit interface.
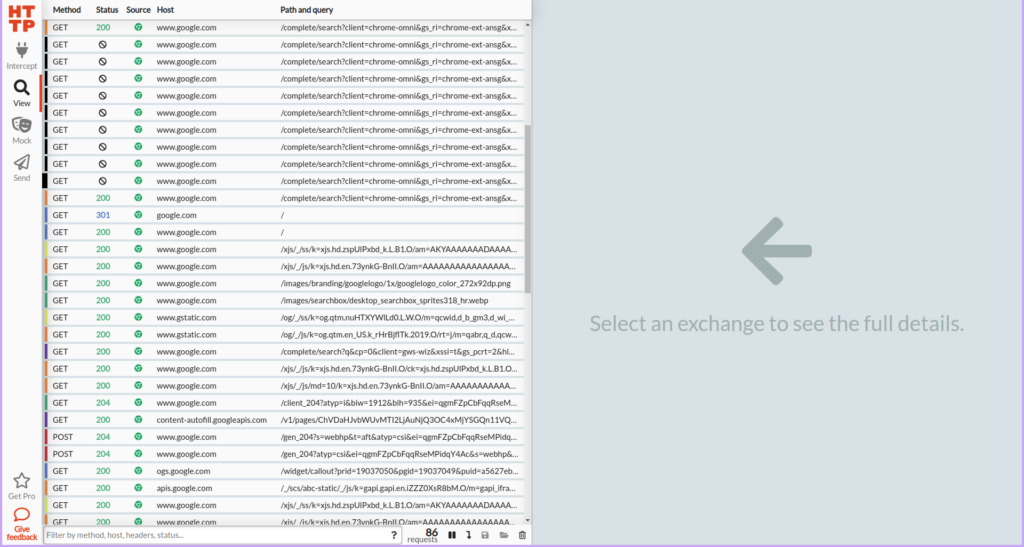
- This feature is particularly useful for debugging web applications, analyzing network traffic, and ensuring that your website is performing optimally. By examining the captured requests, you can gain insights into how data is being transferred between the client and server, identify any issues, and make necessary adjustments.
Charles Proxy
Charles Proxy is an HTTP debugging proxy/application that allows developers to view and analyze all of the HTTP and HTTPS traffic between their machine and the internet. This includes detailed information about requests, responses, and the HTTP headers (which contain the cookies and caching information). By providing a comprehensive view of the data being transferred, it helps developers ensure that their applications are functioning correctly and efficiently. It’s particularly useful for diagnosing issues related to network communication, such as slow load times, broken API calls, or unexpected data. Additionally, Charles Proxy can be used to simulate slower network speeds, test different environments, and even rewrite requests and responses to test various scenarios. This makes it an invaluable tool in the development and debugging process, enhancing the overall quality and performance of web applications.
How to Use Charles Proxy
Charles Proxy is a powerful tool for monitoring and analyzing network traffic. Here is a step-by-step guide to help you get started with using Charles Proxy effectively.
Download and Install:
- To begin, first download the latest version of Charles Proxy that is compatible with your operating system.
- Once the download is complete, proceed with the installation by following the on-screen instructions. This process is straightforward and similar to installing any other software application.
Launch Charles:
- After successfully installing Charles Proxy, locate the application icon and open it. This will launch the Charles Proxy interface, which is where you will be able to monitor and analyze network traffic.
- The first time you run Charles Proxy, you may be prompted to grant certain permissions or configure your network settings. Follow the prompts to ensure everything is set up correctly.
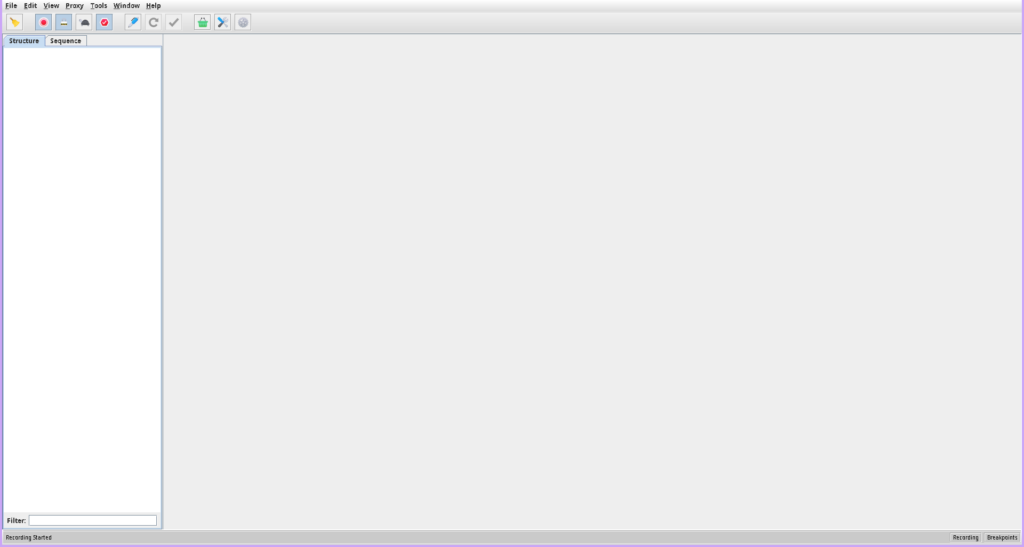
Intercept Network Requests:
- With Charles Proxy running, now launch the application you want to intercept. This could be a web browser, mobile app, or any software that communicates over the network.
- As your application makes network requests, you will see these requests appear in the Charles Proxy interface. This allows you to inspect the details of each request and response, making it easier to debug and analyze network traffic.
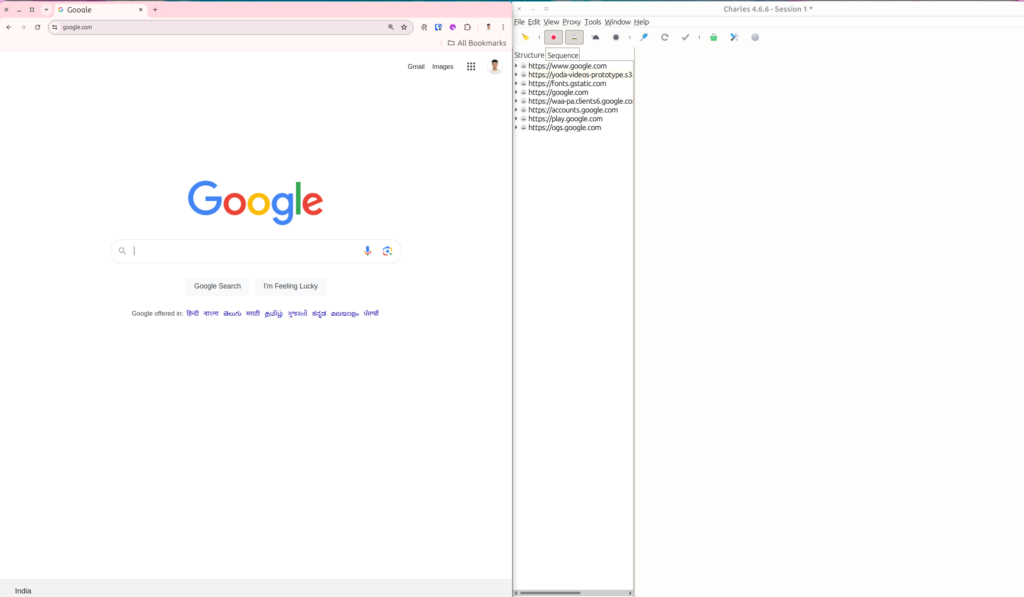
By following these steps, you can effectively use Charles Proxy to monitor and analyze the network requests made by your applications. This tool is invaluable for developers, testers, and anyone needing insight into their application’s network behavior.
Requestly
Requestly is a browser extension that allows you to intercept and modify HTTP requests and responses. It’s useful for testing and debugging web applications.
How to use Requestly :
Requestly is a lightweight, browser-based tool designed for web developers and testers. It allows users to modify network requests and responses on the fly. This includes capabilities like redirecting URLs, modifying headers, and injecting scripts. It’s particularly useful for:
Install Requestly:
- Requestly is available as a browser extension for Chrome, Firefox, and other Chromium-based browsers.
- Download Requestly from it’s official website.
Launch Requestly:
- After installation, click on the Requestly icon in your browser’s toolbar to open the Requestly dashboard.
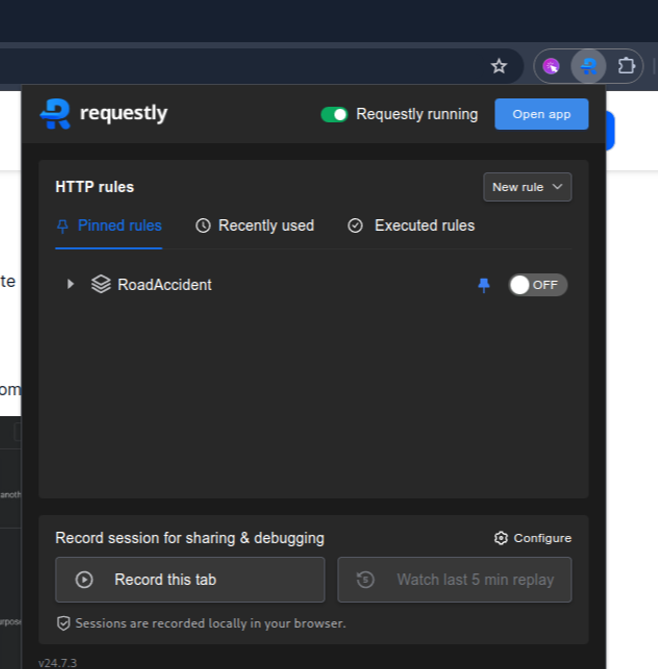
Create a Rule:
- In the dashboard, click on “New Rule” to create a new modification rule.
- Select which type of rule you want to create. Learn about different types of rules from here.
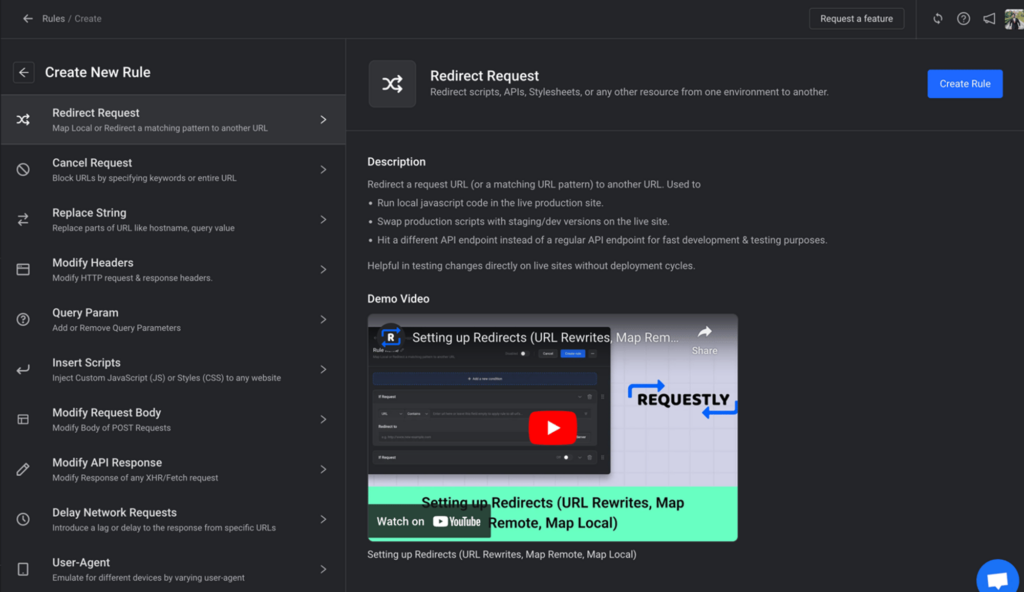
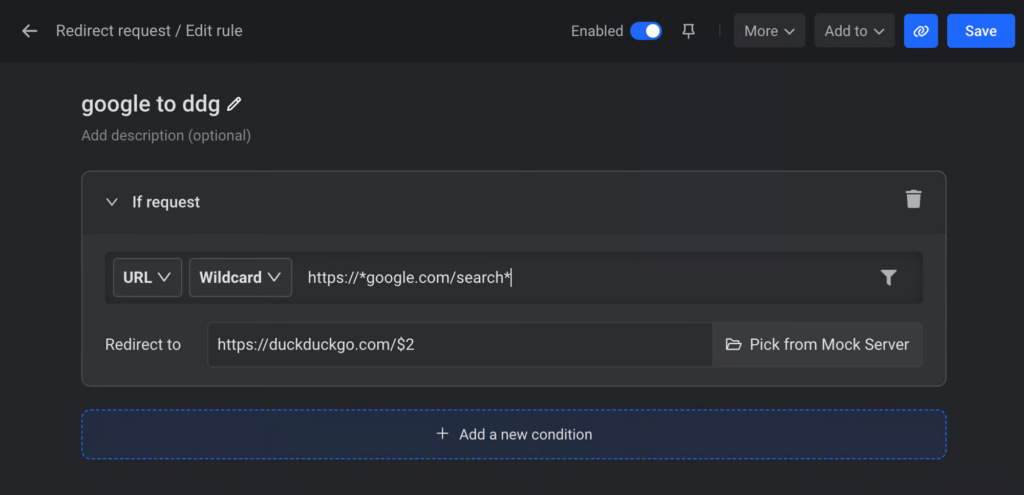
By following these steps, you can effectively use Requestly to manipulate network requests and responses to suit your development and testing needs.
Case Studies
- How Mindera uses Requestly for proactive API Management
- How Bureau.id uses Requestly to toggle staging environment
- How Requestly helps Instabug bridge the gap between frontend and backend
- Streamlining Frontend Development: A Developer’s Journey with Requestly at PingSafe
- How Requestly helps Joyned to test local JS SDK changes directly on customer’s production sites
Recap
In conclusion, HTTP interceptors simplify and enhance web development by centralizing request and response management, improving security, performance, and maintainability. By using tools like Axios, Angular HttpClient, and HTTP Toolkit, developers can efficiently manage client-server communication, leading to more robust applications.
Further Reading
Contents
Subscribe for latest updates
Share this article
Related posts
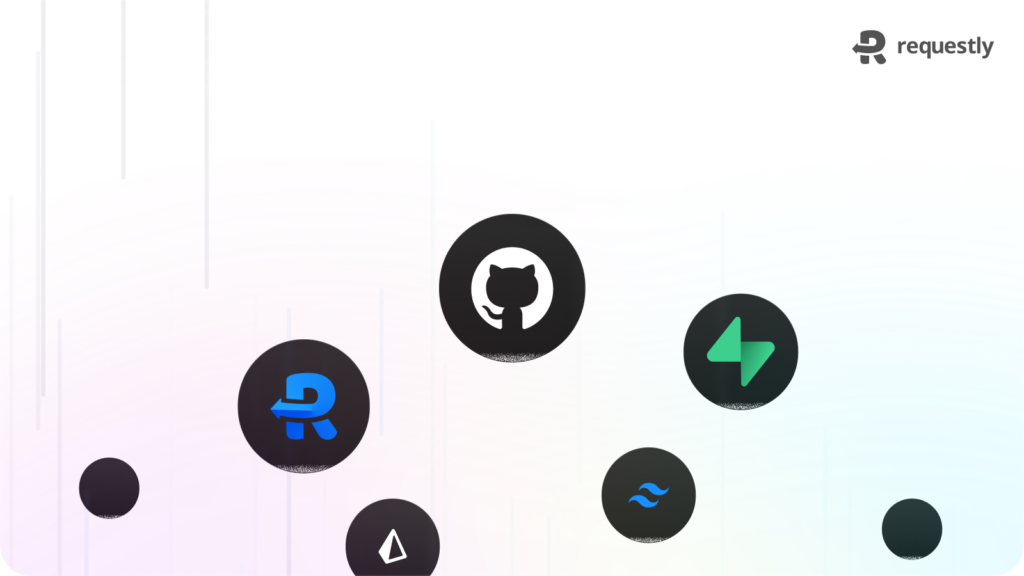
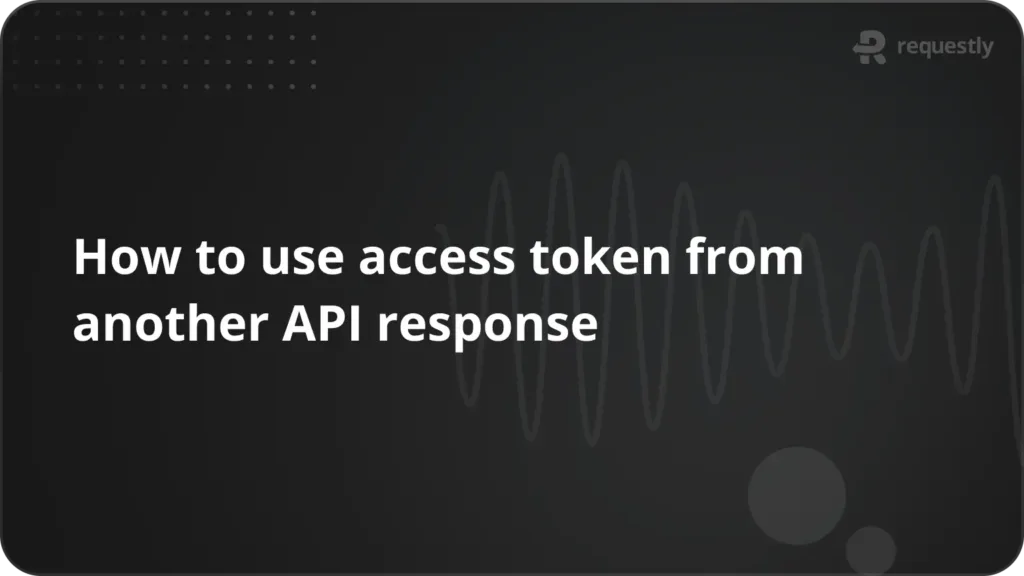
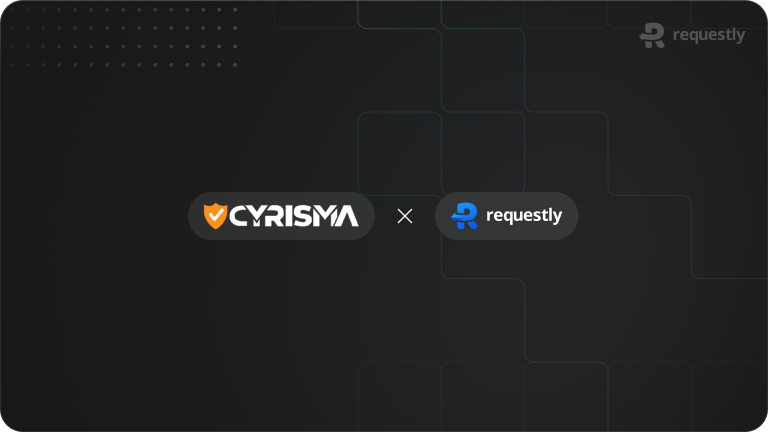